6.2. Communication protocol specification¶
Communication protocol v20.8
- Protocol description
- Command execution
- Controller-side error processing
- Library-side error processing
- Controller error response types
- All controller commands
- Command GACC
- Command GBRK
- Command GCAL
- Command GCTL
- Command GCTP
- Command GEAS
- Command GEDS
- Command GEIO
- Command GEMF
- Command GENG
- Command GENI
- Command GENS
- Command GENT
- Command GEST
- Command GFBS
- Command GGRI
- Command GGRS
- Command GHOM
- Command GHSI
- Command GHSS
- Command GJOY
- Command GMOV
- Command GMTI
- Command GMTS
- Command GNET
- Command GNME
- Command GNMF
- Command GNVM
- Command GPID
- Command GPWD
- Command GPWR
- Command GSEC
- Command GSNI
- Command GSNO
- Command GSTI
- Command GSTS
- Command GURT
- Command SACC
- Command SBRK
- Command SCAL
- Command SCTL
- Command SCTP
- Command SEAS
- Command SEDS
- Command SEIO
- Command SEMF
- Command SENG
- Command SENI
- Command SENS
- Command SENT
- Command SEST
- Command SFBS
- Command SGRI
- Command SGRS
- Command SHOM
- Command SHSI
- Command SHSS
- Command SJOY
- Command SMOV
- Command SMTI
- Command SMTS
- Command SNET
- Command SNME
- Command SNMF
- Command SNVM
- Command SPID
- Command SPWD
- Command SPWR
- Command SSEC
- Command SSNI
- Command SSNO
- Command SSTI
- Command SSTS
- Command SURT
- Command ASIA
- Command CLFR
- Command CONN
- Command DBGR
- Command DBGW
- Command DISC
- Command EERD
- Command EESV
- Command GBLV
- Command GETC
- Command GETI
- Command GETM
- Command GETS
- Command GFWV
- Command GOFW
- Command GPOS
- Command GSER
- Command GUID
- Command HASF
- Command HOME
- Command IRND
- Command LEFT
- Command LOFT
- Command MOVE
- Command MOVR
- Command PWOF
- Command RDAN
- Command READ
- Command RERS
- Command REST
- Command RIGT
- Command SARS
- Command SAVE
- Command SPOS
- Command SSER
- Command SSTP
- Command STMS
- Command STOP
- Command UPDF
- Command WDAT
- Command WKEY
- Command ZERO
6.2.1. Protocol description¶
Controller can be controlled from the PC using serial connection (COM-port). COM-port parameters are fixed controller-side:
- Speed: 115200 baud
- Frame size: 8 bits
- Stop-bits: 2 bits
- Parity: none
- Flow control: none
- Byte receive timeout: 400 ms
- Bit order: little endian
- Byte order: little endian
6.2.2. Command execution¶
All data transfers are initiated by the PC, meaning that the controller waits for incoming commands and replies accordingly. Each command is followed by the controller response, with rare exceptions of some service commands. One should not send another command without waiting for the previous command answer.
Commands are split into service, general control and general information types. Commands are executed immediately. Parameters which are set by Sxxx commands are applied no later than 1ms after acknowledgement. Command processing does not affect real-time engine control (PWM, encoder readout, etc).
Both controller and PC have an IO buffer. Received commands and command data are processed once and then removed from buffer. Each command consists of 4-byte identifier and optionally a data section followed by its 2-byte CRC. Data can be transmitted in both directions, from PC to the controller and vice versa. Command is scheduled for execution if it is a legitimate command and (in case of data) if its CRC matches. After processing a correct command controller replies with 4 bytes - the name of processed command, followed by data and its 2-byte CRC, if the command is supposed to return data.
6.2.3. Controller-side error processing¶
6.2.3.1. Wrong command or data¶
If the controller receives a command that cannot be interpreted as a legitimate command, then controller ignores this command, replies with an “errc” string and sets “command error” flag in the current status data structure. If the unreconized command contained additional data, then it can be interpreted as new command(s). In this case resynchronization is required.
If the controller receives a valid command with data and its CRC doesn’t match the CRC computed by the controller, then controller ignores this command, replies with an “errd” string and sets “data error” flag in the current status data structure. In this case synchronization is not needed.
6.2.3.2. CRC calculation¶
CRC is calculated for data only, 4-byte command identifier is not included. CRC algorithm in C is as follows:
unsigned short CRC16(INT8U *pbuf, unsigned short n)
{
unsigned short crc, i, j, carry_flag, a;
crc = 0xffff;
for(i = 0; i < n; i++)
{
crc = crc ^ pbuf[i];
for(j = 0; j < 8; j++)
{
a = crc;
carry_flag = a & 0x0001;
crc = crc >> 1;
if ( carry_flag == 1 ) crc = crc ^ 0xa001;
}
}
return crc;
}
This function receives a pointer to the data array, pbuf, and data length in bytes, n. It returns a two byte CRC code.
The example of CRC calculation:
Command code (CMD): “home” or 0x656D6F68
0x68 0x6F 0x6D 0x65
CMD
Command code (CMD): “gpos” or 0x736F7067
0x67 0x70 0x6F 0x73
CMD
Command code (CMD): “movr” or 0x72766F6D
0x6D 0x6F 0x76 0x72 0x00 0x00 0x00 0xC8 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x00 0x53 0xc7
CMD DeltaPosition uDPos Reserved CRC
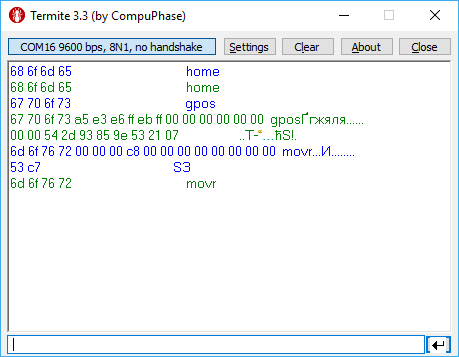
6.2.3.3. Transmission errors¶
Most probable transmission errors are missing, extra or altered byte. In usual settings transmission errors happen rarely, if at all.
Frequent errors are possible when using low-quality or broken USB-cable or board interconnection cable. Protocol is not designed for use in noisy environments and in rare cases an error may match a valid command code and get executed.
Missing byte, controller side “
A missing byte on the controller side leads to a timeout on the PC side. Command is considered to be sent unsuccessfully by the PC. Synchronization is momentarily disrupted and restored after a timeout.
Missing byte, PC side
A missing byte on the PC side leads to a timeout on PC side. Synchronization is maintained.
Extra byte, controller side
An extra byte received by the controller leads to one or several “errc” or “errd” responses. Command is considered to be sent unsuccessfully by the PC. Receive buffer may also contain one or several “errc” or “errd” responses. Synchronization is disrupted.
Extra byte, PC side
An extra byte received by the PC leads to an incorrectly interpreted command or CRC and an extra byte in the receive buffer. Synchronization is disrupted.
Altered byte, controller side
An altered byte received by the controller leads to one or several “errc” or “errd” responses. Command is considered to be sent unsuccessfully by the PC. Receive buffer may also contain one or several “errc” or “errd” responses. Synchronization can rarely be disrupted, but is generally maintained.
Altered byte, PC side
An altered byte received by the PC leads to an incorrectly interpreted command or CRC. Synchronization is maintained.
6.2.3.4. Timeout resynchronization¶
If during packet reception next byte wait time exceeds timeout value, then partially received command is ignored and receive buffer is cleared. Controller timeout should be less than PC timeout, taking into account time it takes to transmit the data.
6.2.3.5. Zero byte resynchronization¶
There are no command codes that start with a zero byte (‘\0’). This allows for a following synchronization procedure: controller always answers with a zero byte if the first command byte is zero, PC ignores first response byte if it is a zero byte. Then, if synchronization is disrupted on either side the following algorithm is used:
In case PC receives “errc”, “errd” or a wrong command answer code, then PC sends 4 to 250 zeroes to the controller (250 byte limit is caused by input buffer length and usage of I2C protocol, less than 4 zeroes do not guarantee successful resynchronization). During this time PC continuously reads incoming bytes from the controller until the first zero is received and stops sending and receiving right after that.
Received zero byte is likely not a part of a response to a previous command because on error PC receives “errc”/”errd” response. It is possible in rare cases, then synchronization procedure will start again. Therefore first zero byte received by the PC means that controller input buffer is already empty and will remain so until any command is sent. Right after receiving first zero byte from the controller PC is ready to transmit next command code. The rest of zero bytes in transit will be ignored because they will be received before controller response.
This completes the zero byte synchronization procedure.
6.2.4. Library-side error processing¶
Nearly every library function has a return status of type result_t.
After sending command to the controller library reads incoming bytes until a non-zero byte is received. All zero bytes are ignored. Library reads first 4 bytes and compares them to the command code. It then waits for data section and CRC, if needed. If first 4 received bytes do not match the sent command identifier, then zero byte synchronization procedure is launched, command is considered to be sent unsuccessfully. If first 4 received bytes match the sent command identifier and command has data section, but the received CRC doesn’t match CRC calculated from the received data, then zero byte synchronization procedure is launched, command is considered to be sent unsuccessfully. If a timeout is reached while the library is waiting for the controller response, then zero byte synchronization procedure is launched, command is considered to be sent unsuccessfully.
If no errors were detected, then command is considered to be successfully completed and result_ok is returned.
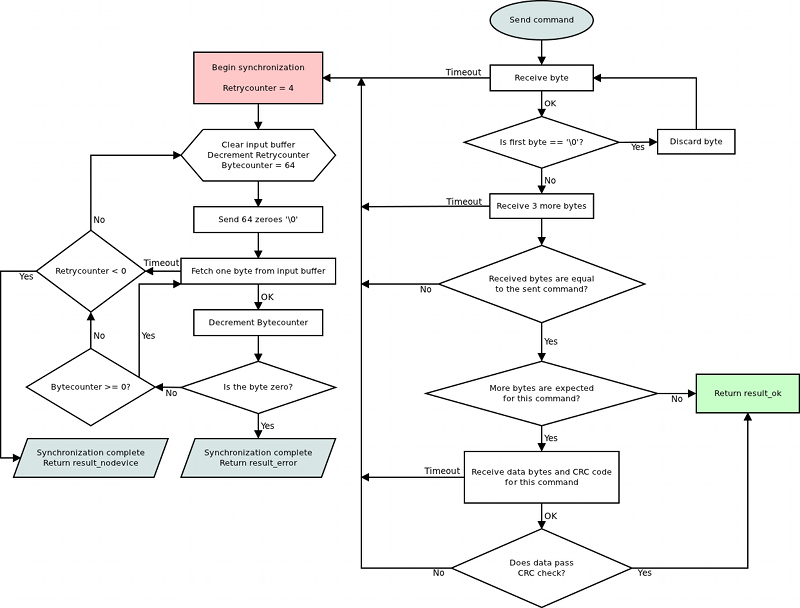
6.2.4.1. Library return codes¶
- result_ok. No errors detected.
- result_error. Generic error. Can happen because of hardware problems, empty port buffer, timeout or successfull synchronization after an error. Another common reason for this error is protocol version mismatch between controller firmware and PC library.
- result_nodevice. Error opening device, lost connection or failed synchronization. Device reopen and/or user action is required.
If a function returns an error values of all parameters it writes to are undefined. Error code may be accompanied by detailed error description output to system log (Unix-like OS) or standard error (Windows-like OS).
6.2.4.2. Zero byte synchronization procedure¶
Synchronization is performed by means of sending zero (‘\0’) bytes and reading bytes until a zero byte is received. Optionally one may clear port buffer at the end of synchronization procedure. Initially 64 zero bytes are sent. If there were no zero bytes received during the timeout, then a string of 64 bytes is sent 3 more times. After 4 unsuccessful attempts and no zero bytes received device is considered lost. In this case library should return result_nodevice error code. In case of successful syncronization library returns result_error.
6.2.5. Controller error response types¶
6.2.5.1. ERRC¶
Answer: (4 bytes)
Code: “errc” or 0x63727265
uint32_t | errc | Command error |
Description:
Controller answers with “errc” if the command is either not recognized or cannot be processed and sets the correspoding bit in status data structure.
6.2.5.2. ERRD¶
Answer: (4 bytes)
Code: “errd” or 0x64727265
uint32_t | errd | Data error |
Description:
Controller answers with “errd” if the CRC of the data section computed by the controller doesn’t match the received CRC field and sets the correspoding bit in status data structure.
6.2.5.3. ERRV¶
Answer: (4 bytes)
Code: “errv” or 0x76727265
uint32_t | errv | Value error |
Description:
Controller answers with “errv” if any of the values in the command are out of acceptable range and can not be applied. Inacceptable value is replaced by a rounded, truncated or default value. Controller also sets the correspoding bit in status data structure.
6.2.6. All controller commands¶
6.2.6.1. Command GACC¶
Command code (CMD): “gacc” or 0x63636167.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (114 bytes)
uint32_t | CMD | Command |
int8_t | MagneticBrakeInfo | The manufacturer and the part number of magnetic brake, the maximum string length is 24 characters. |
float | MBRatedVoltage | Rated voltage for controlling the magnetic brake (V). Data type: float. |
float | MBRatedCurrent | Rated current for controlling the magnetic brake (A). Data type: float. |
float | MBTorque | Retention moment (mN m). Data type: float. |
uint32_t | MBSettings | Flags of magnetic brake settings. This is a bit mask for bitwise operations. |
0x1 - MB_AVAILABLE | If the flag is set, the magnetic brake is available | |
0x2 - MB_POWERED_HOLD | If this flag is set, the magnetic brake is on when powered | |
int8_t | TemperatureSensorInfo | The manufacturer and the part number of the temperature sensor, the maximum string length: 24 characters. |
float | TSMin | The minimum measured temperature (degrees Celsius) Data type: float. |
float | TSMax | The maximum measured temperature (degrees Celsius) Data type: float. |
float | TSGrad | The temperature gradient (V/degrees Celsius). Data type: float. |
uint32_t | TSSettings | Flags of temperature sensor settings. This is a bit mask for bitwise operations. |
0x7 - TS_TYPE_BITS | Bits of the temperature sensor type | |
0x0 - TS_TYPE_UNKNOWN | Unknown type of sensor | |
0x1 - TS_TYPE_THERMOCOUPLE | Thermocouple | |
0x2 - TS_TYPE_SEMICONDUCTOR | The semiconductor temperature sensor | |
0x8 - TS_AVAILABLE | If the flag is set, the temperature sensor is available | |
uint32_t | LimitSwitchesSettings | Flags of limit switches settings. This is a bit mask for bitwise operations. |
0x1 - LS_ON_SW1_AVAILABLE | If the flag is set, the limit switch connected to pin SW1 is available | |
0x2 - LS_ON_SW2_AVAILABLE | If the flag is set, the limit switch connected to pin SW2 is available | |
0x4 - LS_SW1_ACTIVE_LOW | If the flag is set, the limit switch connected to pin SW1 is triggered by a low level on the pin | |
0x8 - LS_SW2_ACTIVE_LOW | If the flag is set, the limit switch connected to pin SW2 is triggered by a low level on pin | |
0x10 - LS_SHORTED | If the flag is set, the limit switches are shorted | |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Description: Deprecated. Read additional accessory information from the EEPROM.
6.2.6.2. Command GBRK¶
Command code (CMD): “gbrk” or 0x6B726267.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (25 bytes)
uint32_t | CMD | Command |
uint16_t | t1 | Time in ms between turning on motor power and turning off the brake. |
uint16_t | t2 | Time in ms between the brake turning off and moving readiness. All moving commands will execute after this interval. |
uint16_t | t3 | Time in ms between motor stop and the brake turning on. |
uint16_t | t4 | Time in ms between turning on the brake and turning off motor power. |
uint8_t | BrakeFlags | Flags. This is a bit mask for bitwise operations. |
0x1 - BRAKE_ENABLED | Brake control is enabled if this flag is set. | |
0x2 - BRAKE_ENG_PWROFF | Brake turns the stepper motor power off if this flag is set. | |
uint8_t | Reserved [10] | Reserved (10 bytes) |
uint16_t | CRC | Checksum |
Description: Read break control settings.
6.2.6.3. Command GCAL¶
Command code (CMD): “gcal” or 0x6C616367.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (118 bytes)
uint32_t | CMD | Command |
float | CSS1_A | Scaling factor for the analog measurements of the A winding current. |
float | CSS1_B | Offset for the analog measurements of the A winding current. |
float | CSS2_A | Scaling factor for the analog measurements of the B winding current. |
float | CSS2_B | Offset for the analog measurements of the B winding current. |
float | FullCurrent_A | Scaling factor for the analog measurements of the full current. |
float | FullCurrent_B | Offset for the analog measurements of the full current. |
uint8_t | Reserved [88] | Reserved (88 bytes) |
uint16_t | CRC | Checksum |
Description: Read calibration settings. Manufacturer only. This function reads the structure with calibration settings. These settings are used to convert bare ADC values to winding currents in mA and the full current in mA. Parameters are grouped into pairs, XXX_A and XXX_B, representing linear equation coefficients. The first one is the slope, the second one is the constant term. Thus, XXX_Current[mA] = XXX_A[mA/ADC]XXX_ADC_CODE[ADC] + XXX_B[mA].
6.2.6.4. Command GCTL¶
Command code (CMD): “gctl” or 0x6C746367.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (93 bytes)
uint32_t | CMD | Command |
uint32_t | MaxSpeed | Array of speeds (full step) used with the joystick and the button control. Range: 0..100000. |
uint8_t | uMaxSpeed | Array of speeds (in microsteps) used with the joystick and the button control. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
uint16_t | Timeout | Timeout[i] is timeout in ms. After that, max_speed[i+1] is applied. It’s used with the button control only. |
uint16_t | MaxClickTime | Maximum click time (in ms). Until the expiration of this time, the first speed isn’t applied. |
uint16_t | Flags | Control flags. This is a bit mask for bitwise operations. |
0x3 - CONTROL_MODE_BITS | Bits to control the engine by joystick or buttons. | |
0x0 - CONTROL_MODE_OFF | Control is disabled. | |
0x1 - CONTROL_MODE_JOY | Control by joystick. | |
0x2 - CONTROL_MODE_LR | Control by left/right buttons. | |
0x4 - CONTROL_BTN_LEFT_PUSHED_OPEN | Pushed left button corresponds to the open contact if this flag is set. | |
0x8 - CONTROL_BTN_RIGHT_PUSHED_OPEN | Pushed right button corresponds to open contact if this flag is set. | |
int32_t | DeltaPosition | Position Shift (delta) (full step) |
int16_t | uDeltaPosition | Fractional part of the shift in micro steps. It’s used with a stepper motor only. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
uint8_t | Reserved [9] | Reserved (9 bytes) |
uint16_t | CRC | Checksum |
Description: Read motor control settings. In case of CTL_MODE=1, joystick motor control is enabled. In this mode, while the joystick is maximally displaced, the engine tends to move at MaxSpeed[i]. i=0 if another value hasn’t been set at the previous usage. To change the speed index ‘i’, use the buttons. In case of CTL_MODE=2, the motor is controlled by the left/right buttons. When you click on the button, the motor starts moving in the appropriate direction at a speed MaxSpeed[0]. After Timeout[i], motor moves at speed MaxSpeed[i+1]. At the transition between MaxSpeed[i] and MaxSpeed[i+1] the motor just accelerates/decelerates as usual.
6.2.6.5. Command GCTP¶
Command code (CMD): “gctp” or 0x70746367.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (18 bytes)
uint32_t | CMD | Command |
uint8_t | CTPMinError | The minimum difference between the SM position in steps and the encoder position that causes the setting of the STATE_CTP_ERROR flag. Measured in steps. |
uint8_t | CTPFlags | This is a bit mask for bitwise operations. |
0x1 - CTP_ENABLED | The position control is enabled if the flag is set. | |
0x2 - CTP_BASE | The position control is based on the revolution sensor if this flag is set; otherwise, it is based on the encoder. | |
0x4 - CTP_ALARM_ON_ERROR | Set ALARM on mismatch if the flag is set. | |
0x8 - REV_SENS_INV | Typically, the sensor is active when it is at 0, and inversion makes active at 1. That is, if you do not invert, it is normal logic - 0 is the activation. | |
0x10 - CTP_ERROR_CORRECTION | Correct errors that appear when slippage occurs if the flag is set. It works only with the encoder. Incompatible with the flag CTP_ALARM_ON_ERROR. | |
uint8_t | Reserved [10] | Reserved (10 bytes) |
uint16_t | CRC | Checksum |
Description: Read control position settings (used with stepper motor only). When controlling the step motor with an encoder (CTP_BASE=0), it is possible to detect the loss of steps. The controller knows the number of steps per revolution (GENG::StepsPerRev) and the encoder resolution (GFBS::IPT). When the control is enabled (CTP_ENABLED is set), the controller stores the current position in the steps of SM and the current position of the encoder. Next, the encoder position is converted into steps at each step, and if the difference between the current position in steps and the encoder position is greater than CTPMinError, the flag STATE_CTP_ERROR is set. Alternatively, the stepper motor may be controlled with the speed sensor (CTP_BASE 1). In this mode, at the active edges of the input clock, the controller stores the current value of steps. Then, at each revolution, the controller checks how many steps have been passed. When the difference is over the CTPMinError, the STATE_CTP_ERROR flag is set.
6.2.6.6. Command GEAS¶
Command code (CMD): “geas” or 0x73616567.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (54 bytes)
uint32_t | CMD | Command |
uint16_t | stepcloseloop_Kw | Mixing ratio of the actual and set speed, range [0, 100], default value 50. |
uint16_t | stepcloseloop_Kp_low | Position feedback in the low-speed zone, range [0, 65535], default value 1000. |
uint16_t | stepcloseloop_Kp_high | Position feedback in the high-speed zone, range [0, 65535], default value 33. |
uint8_t | Reserved [42] | Reserved (42 bytes) |
uint16_t | CRC | Checksum |
Description: Read engine advanced settings.
6.2.6.7. Command GEDS¶
Command code (CMD): “geds” or 0x73646567.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (26 bytes)
uint32_t | CMD | Command |
uint8_t | BorderFlags | Border flags, specify types of borders and motor behavior at borders. This is a bit mask for bitwise operations. |
0x1 - BORDER_IS_ENCODER | Borders are fixed by predetermined encoder values, if set; borders are placed on limit switches, if not set. | |
0x2 - BORDER_STOP_LEFT | The motor should stop on the left border. | |
0x4 - BORDER_STOP_RIGHT | Motor should stop on right border. | |
0x8 - BORDERS_SWAP_MISSET_DETECTION | Motor should stop on both borders. Need to save motor then wrong border settings is set | |
uint8_t | EnderFlags | Flags specify electrical behavior of limit switches like order and pulled positions. This is a bit mask for bitwise operations. |
0x1 - ENDER_SWAP | First limit switch on the right side, if set; otherwise on the left side. | |
0x2 - ENDER_SW1_ACTIVE_LOW | 1 - Limit switch connected to pin SW1 is triggered by a low level on pin. | |
0x4 - ENDER_SW2_ACTIVE_LOW | 1 - Limit switch connected to pin SW2 is triggered by a low level on pin. | |
int32_t | LeftBorder | Left border position, used if BORDER_IS_ENCODER flag is set. |
int16_t | uLeftBorder | Left border position in microsteps (used with stepper motor only). The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
int32_t | RightBorder | Right border position, used if BORDER_IS_ENCODER flag is set. |
int16_t | uRightBorder | Right border position in microsteps. Used with a stepper motor only. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
uint8_t | Reserved [6] | Reserved (6 bytes) |
uint16_t | CRC | Checksum |
Description: Read border and limit switches settings.
6.2.6.8. Command GEIO¶
Command code (CMD): “geio” or 0x6F696567.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (18 bytes)
uint32_t | CMD | Command |
uint8_t | EXTIOSetupFlags | Configuration flags of the external I-O. This is a bit mask for bitwise operations. |
0x1 - EXTIO_SETUP_OUTPUT | EXTIO works as output if the flag is set, works as input otherwise. | |
0x2 - EXTIO_SETUP_INVERT | Interpret EXTIO state inverted if the flag is set. A falling front is treated as an input event and a low logic level as an active state. | |
uint8_t | EXTIOModeFlags | Flags mode settings external I-O. This is a bit mask for bitwise operations. |
0xf - EXTIO_SETUP_MODE_IN_BITS | Bits of the behavior selector when the signal on input goes to the active state. | |
0x0 - EXTIO_SETUP_MODE_IN_NOP | Do nothing. | |
0x1 - EXTIO_SETUP_MODE_IN_STOP | Issue STOP command, ceasing the engine movement. | |
0x2 - EXTIO_SETUP_MODE_IN_PWOF | Issue PWOF command, powering off all engine windings. | |
0x3 - EXTIO_SETUP_MODE_IN_MOVR | Issue MOVR command with last used settings. | |
0x4 - EXTIO_SETUP_MODE_IN_HOME | Issue HOME command. | |
0x5 - EXTIO_SETUP_MODE_IN_ALARM | Set Alarm when the signal goes to the active state. | |
0xf0 - EXTIO_SETUP_MODE_OUT_BITS | Bits of the output behavior selection. | |
0x0 - EXTIO_SETUP_MODE_OUT_OFF | EXTIO pin always set in inactive state. | |
0x10 - EXTIO_SETUP_MODE_OUT_ON | EXTIO pin always set in active state. | |
0x20 - EXTIO_SETUP_MODE_OUT_MOVING | EXTIO pin stays active during moving state. | |
0x30 - EXTIO_SETUP_MODE_OUT_ALARM | EXTIO pin stays active during the alarm state. | |
0x40 - EXTIO_SETUP_MODE_OUT_MOTOR_ON | EXTIO pin stays active when windings are powered. | |
uint8_t | Reserved [10] | Reserved (10 bytes) |
uint16_t | CRC | Checksum |
Description: Read EXTIO settings. This function reads a structure with a set of EXTIO settings from the controller’s memory.
6.2.6.9. Command GEMF¶
Command code (CMD): “gemf” or 0x666D6567.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (48 bytes)
uint32_t | CMD | Command |
float | L | Motor winding inductance. |
float | R | Motor winding resistance. |
float | Km | Electromechanical ratio of the motor. |
uint8_t | BackEMFFlags | Auto-settings stepper motor flags. This is a bit mask for bitwise operations. |
0x1 - BACK_EMF_INDUCTANCE_AUTO | Flag of auto-detection of inductance of windings of the engine. | |
0x2 - BACK_EMF_RESISTANCE_AUTO | Flag of auto-detection of resistance of windings of the engine. | |
0x4 - BACK_EMF_KM_AUTO | Flag of auto-detection of electromechanical coefficient of the engine. | |
uint8_t | Reserved [29] | Reserved (29 bytes) |
uint16_t | CRC | Checksum |
Description: Read electromechanical settings. The settings are different for different stepper motors.
6.2.6.10. Command GENG¶
Command code (CMD): “geng” or 0x676E6567.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (34 bytes)
uint32_t | CMD | Command |
uint16_t | NomVoltage | Rated voltage in tens of mV. Controller will keep the voltage drop on motor below this value if ENGINE_LIMIT_VOLT flag is set (used with DC only). |
uint16_t | NomCurrent | Rated current (in mA). Controller will keep current consumed by motor below this value if ENGINE_LIMIT_CURR flag is set. Range: 15..8000 |
uint32_t | NomSpeed | Nominal (maximum) speed (in whole steps/s or rpm for DC and stepper motor as a master encoder). Controller will keep motor shaft RPM below this value if ENGINE_LIMIT_RPM flag is set. Range: 1..100000. |
uint8_t | uNomSpeed | The fractional part of a nominal speed in microsteps (is only used with stepper motor). Microstep size and the range of valid values for this field depend on selected step division mode (see MicrostepMode field in engine_settings). |
uint16_t | EngineFlags | Set of flags specify motor shaft movement algorithm and a list of limitations. This is a bit mask for bitwise operations. |
0x1 - ENGINE_REVERSE | Reverse flag. It determines motor shaft rotation direction that corresponds to feedback counts increasing. If not set (default), motor shaft rotation direction under positive voltage corresponds to feedback counts increasing and vice versa. Change it if you see that positive directions on motor and feedback are opposite. | |
0x2 - ENGINE_CURRENT_AS_RMS | Engine current meaning flag. If the flag is unset, then the engine’s current value is interpreted as the maximum amplitude value. If the flag is set, then the engine current value is interpreted as the root-mean-square current value (for stepper) or as the current value calculated from the maximum heat dissipation (BLDC). | |
0x4 - ENGINE_MAX_SPEED | Max speed flag. If it is set, the engine uses the maximum speed achievable with the present engine settings as its nominal speed. | |
0x8 - ENGINE_ANTIPLAY | Play compensation flag. If it is set, the engine makes backlash (play) compensation and reaches the predetermined position accurately at low speed. | |
0x10 - ENGINE_ACCEL_ON | Acceleration enable flag. If it set, motion begins with acceleration and ends with deceleration. | |
0x20 - ENGINE_LIMIT_VOLT | Maximum motor voltage limit enable flag (is only used with DC motor). | |
0x40 - ENGINE_LIMIT_CURR | Maximum motor current limit enable flag (is only used with DC motor). | |
0x80 - ENGINE_LIMIT_RPM | Maximum motor speed limit enable flag. | |
int16_t | Antiplay | Number of pulses or steps for backlash (play) compensation procedure. Used if ENGINE_ANTIPLAY flag is set. |
uint8_t | MicrostepMode | Settings of microstep mode (Used with stepper motor only). the microstep size and the range of valid values for this field depend on the selected step division mode (see MicrostepMode field in engine_settings). This is a bit mask for bitwise operations. |
0x1 - MICROSTEP_MODE_FULL | Full step mode. | |
0x2 - MICROSTEP_MODE_FRAC_2 | 1/2-step mode. | |
0x3 - MICROSTEP_MODE_FRAC_4 | 1/4-step mode. | |
0x4 - MICROSTEP_MODE_FRAC_8 | 1/8-step mode. | |
0x5 - MICROSTEP_MODE_FRAC_16 | 1/16-step mode. | |
0x6 - MICROSTEP_MODE_FRAC_32 | 1/32-step mode. | |
0x7 - MICROSTEP_MODE_FRAC_64 | 1/64-step mode. | |
0x8 - MICROSTEP_MODE_FRAC_128 | 1/128-step mode. | |
0x9 - MICROSTEP_MODE_FRAC_256 | 1/256-step mode. | |
uint16_t | StepsPerRev | Number of full steps per revolution (Used with stepper motor only). Range: 1..65535. |
uint8_t | Reserved [12] | Reserved (12 bytes) |
uint16_t | CRC | Checksum |
Description: Read engine settings. This function reads the structure containing a set of useful motor settings stored in the controller’s memory. These settings specify motor shaft movement algorithm, list of limitations and rated characteristics.
6.2.6.11. Command GENI¶
Command code (CMD): “geni” or 0x696E6567.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (70 bytes)
uint32_t | CMD | Command |
int8_t | Manufacturer | Manufacturer. Max string length: 16 chars. |
int8_t | PartNumber | Series and PartNumber. Max string length: 24 chars. |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Description: Deprecated. Read encoder information from the EEPROM.
6.2.6.12. Command GENS¶
Command code (CMD): “gens” or 0x736E6567.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (54 bytes)
uint32_t | CMD | Command |
float | MaxOperatingFrequency | Maximum operation frequency (kHz). Data type: float. |
float | SupplyVoltageMin | Minimum supply voltage (V). Data type: float. |
float | SupplyVoltageMax | Maximum supply voltage (V). Data type: float. |
float | MaxCurrentConsumption | Max current consumption (mA). Data type: float. |
uint32_t | PPR | The number of counts per revolution |
uint32_t | EncoderSettings | Encoder settings flags. This is a bit mask for bitwise operations. |
0x1 - ENCSET_DIFFERENTIAL_OUTPUT | If the flag is set, the encoder has differential output, otherwise single-ended output | |
0x4 - ENCSET_PUSHPULL_OUTPUT | If the flag is set the encoder has push-pull output, otherwise open drain output | |
0x10 - ENCSET_INDEXCHANNEL_PRESENT | If the flag is set, the encoder has an extra indexed channel | |
0x40 - ENCSET_REVOLUTIONSENSOR_PRESENT | If the flag is set, the encoder has the revolution sensor | |
0x100 - ENCSET_REVOLUTIONSENSOR_ACTIVE_HIGH | If the flag is set, the revolution sensor’s active state is high logic state; otherwise, the active state is low logic state | |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Description: Deprecated. Read encoder settings from the EEPROM.
6.2.6.13. Command GENT¶
Command code (CMD): “gent” or 0x746E6567.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (14 bytes)
uint32_t | CMD | Command |
uint8_t | EngineType | Engine type. This is a bit mask for bitwise operations. |
0x0 - ENGINE_TYPE_NONE | A value that shouldn’t be used. | |
0x1 - ENGINE_TYPE_DC | DC motor. | |
0x2 - ENGINE_TYPE_2DC | 2 DC motors. | |
0x3 - ENGINE_TYPE_STEP | Step motor. | |
0x4 - ENGINE_TYPE_TEST | Duty cycle are fixed. Used only manufacturer. | |
0x5 - ENGINE_TYPE_BRUSHLESS | Brushless motor. | |
uint8_t | DriverType | Driver type. This is a bit mask for bitwise operations. |
0x1 - DRIVER_TYPE_DISCRETE_FET | Driver with discrete FET keys. Default option. | |
0x2 - DRIVER_TYPE_INTEGRATE | Driver with integrated IC. | |
0x3 - DRIVER_TYPE_EXTERNAL | External driver. | |
uint8_t | Reserved [6] | Reserved (6 bytes) |
uint16_t | CRC | Checksum |
Description: Return engine type and driver type.
6.2.6.14. Command GEST¶
Command code (CMD): “gest” or 0x74736567.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (46 bytes)
uint32_t | CMD | Command |
uint16_t | Param1 | |
uint8_t | Reserved [38] | Reserved (38 bytes) |
uint16_t | CRC | Checksum |
Description: Read extended settings. Currently, it is not in use.
6.2.6.15. Command GFBS¶
Command code (CMD): “gfbs” or 0x73626667.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (18 bytes)
uint32_t | CMD | Command |
uint16_t | IPS | The number of encoder counts per shaft revolution. Range: 1..655535. The field is obsolete, it is recommended to write 0 to IPS and use the extended CountsPerTurn field. You may need to update the controller firmware to the latest version. |
uint8_t | FeedbackType | Type of feedback. This is a bit mask for bitwise operations. |
0x1 - FEEDBACK_ENCODER | Feedback by encoder. | |
0x4 - FEEDBACK_EMF | Feedback by EMF. | |
0x5 - FEEDBACK_NONE | Feedback is absent. | |
0x6 - FEEDBACK_ENCODER_MEDIATED | Feedback by encoder mediated by mechanical transmission (for example leadscrew). | |
uint8_t | FeedbackFlags | Flags. This is a bit mask for bitwise operations. |
0x1 - FEEDBACK_ENC_REVERSE | Reverse count of encoder. | |
0xc0 - FEEDBACK_ENC_TYPE_BITS | Bits of the encoder type. | |
0x0 - FEEDBACK_ENC_TYPE_AUTO | Auto detect encoder type. | |
0x40 - FEEDBACK_ENC_TYPE_SINGLE_ENDED | Single-ended encoder. | |
0x80 - FEEDBACK_ENC_TYPE_DIFFERENTIAL | Differential encoder. | |
uint32_t | CountsPerTurn | The number of encoder counts per shaft revolution. Range: 1..4294967295. To use the CountsPerTurn field, write 0 in the IPS field, otherwise the value from the IPS field will be used. |
uint8_t | Reserved [4] | Reserved (4 bytes) |
uint16_t | CRC | Checksum |
Description: Feedback settings.
6.2.6.16. Command GGRI¶
Command code (CMD): “ggri” or 0x69726767.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (70 bytes)
uint32_t | CMD | Command |
int8_t | Manufacturer | Manufacturer. Max string length: 16 chars. |
int8_t | PartNumber | Series and PartNumber. Max string length: 24 chars. |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Description: Deprecated. Read gear information from the EEPROM.
6.2.6.17. Command GGRS¶
Command code (CMD): “ggrs” or 0x73726767.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (58 bytes)
uint32_t | CMD | Command |
float | ReductionIn | Input reduction coefficient. (Output = (ReductionOut / ReductionIn) Input) Data type: float. |
float | ReductionOut | Output reduction coefficient. (Output = (ReductionOut / ReductionIn) Input) Data type: float. |
float | RatedInputTorque | Maximum continuous torque (N m). Data type: float. |
float | RatedInputSpeed | Maximum speed on the input shaft (rpm). Data type: float. |
float | MaxOutputBacklash | Output backlash of the reduction gear (degree). Data type: float. |
float | InputInertia | Equivalent input gear inertia (g cm2). Data type: float. |
float | Efficiency | Reduction gear efficiency (%). Data type: float. |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Description: Deprecated. Read gear settings from the EEPROM.
6.2.6.18. Command GHOM¶
Command code (CMD): “ghom” or 0x6D6F6867.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (33 bytes)
uint32_t | CMD | Command |
uint32_t | FastHome | Speed used for first motion (full steps). Range: 0..100000. |
uint8_t | uFastHome | Fractional part of the speed for first motion, microsteps. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
uint32_t | SlowHome | Speed used for second motion (full steps). Range: 0..100000. |
uint8_t | uSlowHome | Part of the speed for second motion, microsteps. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
int32_t | HomeDelta | Distance from break point (full steps). |
int16_t | uHomeDelta | Fractional part of the delta distance, microsteps. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
uint16_t | HomeFlags | Set of flags specifies the direction and stopping conditions. This is a bit mask for bitwise operations. |
0x1 - HOME_DIR_FIRST | The flag defines the direction of the 1st motion after execution of the home command. The direction is to the right if the flag is set, and to the left otherwise. | |
0x2 - HOME_DIR_SECOND | The flag defines the direction of the 2nd motion. The direction is to the right if the flag is set, and to the left otherwise. | |
0x4 - HOME_MV_SEC_EN | Use the second phase of calibration to the home position, if set; otherwise the second phase is skipped. | |
0x8 - HOME_HALF_MV | If the flag is set, the stop signals are ignored during the first half-turn of the second movement. | |
0x30 - HOME_STOP_FIRST_BITS | Bits of the first stop selector. | |
0x10 - HOME_STOP_FIRST_REV | First motion stops by revolution sensor. | |
0x20 - HOME_STOP_FIRST_SYN | First motion stops by synchronization input. | |
0x30 - HOME_STOP_FIRST_LIM | First motion stops by limit switch. | |
0xc0 - HOME_STOP_SECOND_BITS | Bits of the second stop selector. | |
0x40 - HOME_STOP_SECOND_REV | Second motion stops by revolution sensor. | |
0x80 - HOME_STOP_SECOND_SYN | Second motion stops by synchronization input. | |
0xc0 - HOME_STOP_SECOND_LIM | Second motion stops by limit switch. | |
0x100 - HOME_USE_FAST | Use the fast algorithm of calibration to the home position, if set; otherwise the traditional algorithm. | |
uint8_t | Reserved [9] | Reserved (9 bytes) |
uint16_t | CRC | Checksum |
Description: Read home settings. This function reads the structure with home position settings.
6.2.6.19. Command GHSI¶
Command code (CMD): “ghsi” or 0x69736867.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (70 bytes)
uint32_t | CMD | Command |
int8_t | Manufacturer | Manufacturer. Max string length: 16 chars. |
int8_t | PartNumber | Series and PartNumber. Max string length: 24 chars. |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Description: Deprecated. Read hall sensor information from the EEPROM.
6.2.6.20. Command GHSS¶
Command code (CMD): “ghss” or 0x73736867.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (50 bytes)
uint32_t | CMD | Command |
float | MaxOperatingFrequency | Maximum operation frequency (kHz). Data type: float. |
float | SupplyVoltageMin | Minimum supply voltage (V). Data type: float. |
float | SupplyVoltageMax | Maximum supply voltage (V). Data type: float. |
float | MaxCurrentConsumption | Maximum current consumption (mA). Data type: float. |
uint32_t | PPR | The number of counts per revolution |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Description: Deprecated. Read hall sensor settings from the EEPROM.
6.2.6.21. Command GJOY¶
Command code (CMD): “gjoy” or 0x796F6A67.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (22 bytes)
uint32_t | CMD | Command |
uint16_t | JoyLowEnd | Joystick lower end position. Range: 0..10000. |
uint16_t | JoyCenter | Joystick center position. Range: 0..10000. |
uint16_t | JoyHighEnd | Joystick upper end position. Range: 0..10000. |
uint8_t | ExpFactor | Exponential nonlinearity factor. |
uint8_t | DeadZone | Joystick dead zone. |
uint8_t | JoyFlags | Joystick control flags. This is a bit mask for bitwise operations. |
0x1 - JOY_REVERSE | Joystick action is reversed. The joystick deviation to the upper values corresponds to negative speed and vice versa. | |
uint8_t | Reserved [7] | Reserved (7 bytes) |
uint16_t | CRC | Checksum |
Description: Read joystick settings. If joystick position falls outside DeadZone limits, a movement begins. The speed is defined by the joystick’s position in the range of the DeadZone limit to the maximum deviation. Joystick positions inside DeadZone limits correspond to zero speed (a soft stop of the motion), and positions beyond Low and High limits correspond to MaxSpeed[i] or -MaxSpeed[i] (see command SCTL), where i = 0 by default and can be changed with the left/right buttons (see command SCTL). If the next speed in the list is zero (both integer and microstep parts), the button press is ignored. The first speed in the list shouldn’t be zero. The DeadZone ranges are illustrated on the following picture.
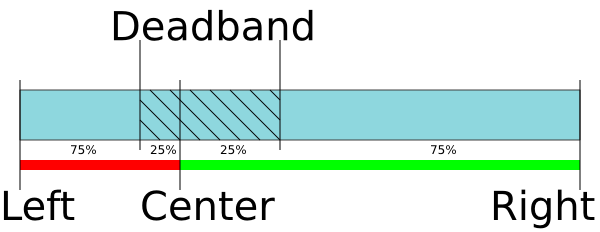
The relationship between the deviation and the rate is exponential, allowing no switching speed combine high mobility and accuracy. The following picture illustrates this:
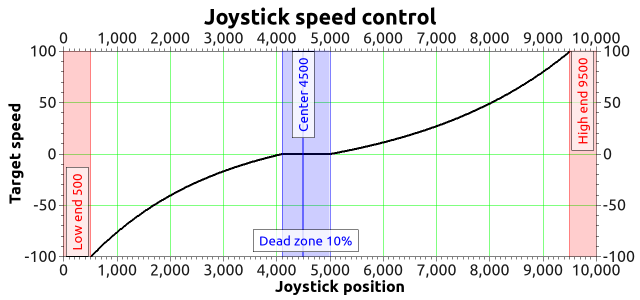
The nonlinearity parameter is adjustable. Setting it to zero makes deviation/speed relation linear.
6.2.6.22. Command GMOV¶
Command code (CMD): “gmov” or 0x766F6D67.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (30 bytes)
uint32_t | CMD | Command |
uint32_t | Speed | Target speed (for stepper motor: steps/s, for DC: rpm). Range: 0..100000. |
uint8_t | uSpeed | Target speed in microstep fractions/s. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). Used with a stepper motor only. |
uint16_t | Accel | Motor shaft acceleration, steps/s^2 (stepper motor) or RPM/s (DC). Range: 1..65535. |
uint16_t | Decel | Motor shaft deceleration, steps/s^2 (stepper motor) or RPM/s (DC). Range: 1..65535. |
uint32_t | AntiplaySpeed | Speed in antiplay mode, full steps/s (stepper motor) or RPM (DC). Range: 0..100000. |
uint8_t | uAntiplaySpeed | Speed in antiplay mode, microsteps/s. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). Used with a stepper motor only. |
uint8_t | MoveFlags | Flags that control movement settings. This is a bit mask for bitwise operations. |
0x1 - RPM_DIV_1000 | This flag indicates that the operating speed specified in the command is set in milliRPM. Applicable only for ENCODER feedback mode and only for BLDC motors. | |
uint8_t | Reserved [9] | Reserved (9 bytes) |
uint16_t | CRC | Checksum |
Description: Movement settings read command (speed, acceleration, threshold, etc.).
6.2.6.23. Command GMTI¶
Command code (CMD): “gmti” or 0x69746D67.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (70 bytes)
uint32_t | CMD | Command |
int8_t | Manufacturer | Manufacturer. Max string length: 16 chars. |
int8_t | PartNumber | Series and PartNumber. Max string length: 24 chars. |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Description: Deprecated. Read motor information from the EEPROM.
6.2.6.24. Command GMTS¶
Command code (CMD): “gmts” or 0x73746D67.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (112 bytes)
uint32_t | CMD | Command |
uint8_t | MotorType | Motor type. This is a bit mask for bitwise operations. |
0x0 - MOTOR_TYPE_UNKNOWN | Unknown type of engine | |
0x1 - MOTOR_TYPE_STEP | Step engine | |
0x2 - MOTOR_TYPE_DC | DC engine | |
0x3 - MOTOR_TYPE_BLDC | BLDC engine | |
uint8_t | ReservedField | Reserved |
uint16_t | Poles | Number of pole pairs for DC or BLDC motors or number of steps per rotation for stepper motors. |
uint16_t | Phases | Number of phases for BLDC motors. |
float | NominalVoltage | Nominal voltage on winding (B). Data type: float |
float | NominalCurrent | Maximum direct current in winding for DC and BLDC engines, nominal current in windings for stepper motors (A). Data type: float. |
float | NominalSpeed | Not used. Nominal speed (rpm). Used for DC and BLDC engines. Data type: float. |
float | NominalTorque | Nominal torque (mN m). Used for DC and BLDC engines. Data type: float. |
float | NominalPower | Nominal power (W). Used for DC and BLDC engines. Data type: float. |
float | WindingResistance | Resistance of windings for DC engines, of each of two windings for stepper motors, or of each of three windings for BLDC engines (Ohm). Data type: float. |
float | WindingInductance | Inductance of windings for DC engines, inductance of each of two windings for stepper motors, or inductance of each of three windings for BLDC engines (mH). Data type: float. |
float | RotorInertia | Rotor inertia (g cm2). Data type: float. |
float | StallTorque | Torque hold position for a stepper motor or torque at a motionless rotor for other types of engines (mN m). Data type: float. |
float | DetentTorque | Holding torque position with unpowered windings (mN m). Data type: float. |
float | TorqueConstant | Torque constant that determines the proportionality constant between the maximum rotor torque and current flowing in the winding (mN m / A). Used mainly for DC motors. Data type: float. |
float | SpeedConstant | Velocity constant, which determines the value or the amplitude of the induced voltage on the motion of DC or BLDC motors (rpm / V) or stepper motors (steps/s / V). Data type: float. |
float | SpeedTorqueGradient | Speed torque gradient (rpm / mN m). Data type: float. |
float | MechanicalTimeConstant | Mechanical time constant (ms). Data type: float. |
float | MaxSpeed | The maximum speed for stepper motors (steps/s) or DC and BLDC motors (rmp). Data type: float. |
float | MaxCurrent | The maximum current in the winding (A). Data type: float. |
float | MaxCurrentTime | Safe duration of overcurrent in the winding (ms). Data type: float. |
float | NoLoadCurrent | The current consumption in idle mode (A). Used for DC and BLDC motors. Data type: float. |
float | NoLoadSpeed | Idle speed (rpm). Used for DC and BLDC motors. Data type: float. |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Description: Deprecated. Read motor settings from the EEPROM.
6.2.6.25. Command GNET¶
Command code (CMD): “gnet” or 0x74656E67.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (38 bytes)
uint32_t | CMD | Command |
uint8_t | DHCPEnabled | Indicates the method to get the IP-address. It can be either 0 (static) or 1 (DHCP). |
uint8_t | IPv4Address | IP-address of the device in format x.x.x.x. |
uint8_t | SubnetMask | The mask of the subnet in format x.x.x.x. |
uint8_t | DefaultGateway | Default value of the gateway in format x.x.x.x. |
uint8_t | Reserved [19] | Reserved (19 bytes) |
uint16_t | CRC | Checksum |
Description: Read network settings. Manufacturer only. This function returns the current network settings.
6.2.6.26. Command GNME¶
Command code (CMD): “gnme” or 0x656D6E67.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (30 bytes)
uint32_t | CMD | Command |
int8_t | PositionerName | User’s positioner name. It can be set by a user. Max string length: 16 characters. |
uint8_t | Reserved [8] | Reserved (8 bytes) |
uint16_t | CRC | Checksum |
Description: Read the user’s stage name from the EEPROM.
6.2.6.27. Command GNMF¶
Command code (CMD): “gnmf” or 0x666D6E67.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (30 bytes)
uint32_t | CMD | Command |
int8_t | ControllerName | User controller name. It may be set by the user. Max string length: 16 characters. |
uint8_t | CtrlFlags | Internal controller settings. This is a bit mask for bitwise operations. |
0x1 - EEPROM_PRECEDENCE | If the flag is set, settings from external EEPROM override controller settings. | |
uint8_t | Reserved [7] | Reserved (7 bytes) |
uint16_t | CRC | Checksum |
Description: Read user’s controller name and internal settings from the FRAM.
6.2.6.28. Command GNVM¶
Command code (CMD): “gnvm” or 0x6D766E67.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (36 bytes)
uint32_t | CMD | Command |
uint32_t | UserData | User data. It may be set by the user. Each element of the array stores only 32 bits of user data. This is important on systems where an int type contains more than 4 bytes. For example, on all amd64 systems. |
uint8_t | Reserved [2] | Reserved (2 bytes) |
uint16_t | CRC | Checksum |
Description: Read user data from FRAM.
6.2.6.29. Command GPID¶
Command code (CMD): “gpid” or 0x64697067.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (48 bytes)
uint32_t | CMD | Command |
uint16_t | KpU | Proportional gain for voltage PID routine |
uint16_t | KiU | Integral gain for voltage PID routine |
uint16_t | KdU | Differential gain for voltage PID routine |
float | Kpf | Proportional gain for BLDC position PID routine |
float | Kif | Integral gain for BLDC position PID routine |
float | Kdf | Differential gain for BLDC position PID routine |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Description: Read PID settings. This function reads the structure containing a set of motor PID settings stored in the controller’s memory. These settings specify the behavior of the PID routine for the positioner. These factors are slightly different for different positioners. All boards are supplied with the standard set of PID settings in the controller’s flash memory.
6.2.6.30. Command GPWD¶
Command code (CMD): “gpwd” or 0x64777067.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (36 bytes)
uint32_t | CMD | Command |
int8_t | UserPassword | Password for the web-page that the user can change with a USB command or via web-page. |
uint8_t | Reserved [10] | Reserved (10 bytes) |
uint16_t | CRC | Checksum |
Description: Read the password. Manufacturer only. This function reads the user password for the device’s web-page.
6.2.6.31. Command GPWR¶
Command code (CMD): “gpwr” or 0x72777067.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (20 bytes)
uint32_t | CMD | Command |
uint8_t | HoldCurrent | Holding current, as percent of the nominal current. Range: 0..100. |
uint16_t | CurrReductDelay | Time in ms from going to STOP state to the end of current reduction. |
uint16_t | PowerOffDelay | Time in s from going to STOP state to turning power off. |
uint16_t | CurrentSetTime | Time in ms to reach the nominal current. |
uint8_t | PowerFlags | Flags with parameters of power control. This is a bit mask for bitwise operations. |
0x1 - POWER_REDUCT_ENABLED | Current reduction is enabled after CurrReductDelay if this flag is set. | |
0x2 - POWER_OFF_ENABLED | Power off is enabled after PowerOffDelay if this flag is set. | |
0x4 - POWER_SMOOTH_CURRENT | Current ramp-up/down are performed smoothly during current_set_time if this flag is set. | |
uint8_t | Reserved [6] | Reserved (6 bytes) |
uint16_t | CRC | Checksum |
Description: Read settings of step motor power control. Used with a stepper motor only.
6.2.6.32. Command GSEC¶
Command code (CMD): “gsec” or 0x63657367.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (28 bytes)
uint32_t | CMD | Command |
uint16_t | LowUpwrOff | Lower voltage limit to turn off the motor, in tens of mV. |
uint16_t | CriticalIpwr | Maximum motor current which triggers ALARM state, in mA. |
uint16_t | CriticalUpwr | Maximum motor voltage which triggers ALARM state, in tens of mV. |
uint16_t | CriticalT | Maximum temperature, which triggers ALARM state, in tenths of degrees Celsius. |
uint16_t | CriticalIusb | Maximum USB current which triggers ALARM state, in mA. |
uint16_t | CriticalUusb | Maximum USB voltage which triggers ALARM state, in tens of mV. |
uint16_t | MinimumUusb | Minimum USB voltage which triggers ALARM state, in tens of mV. |
uint8_t | Flags | Critical parameter flags. This is a bit mask for bitwise operations. |
0x1 - ALARM_ON_DRIVER_OVERHEATING | If this flag is set, enter the alarm state on the driver overheat signal. | |
0x2 - LOW_UPWR_PROTECTION | If this flag is set, turn off the motor when the voltage is lower than LowUpwrOff. | |
0x4 - H_BRIDGE_ALERT | If this flag is set then turn off the power unit with a signal problem in one of the transistor bridge. | |
0x8 - ALARM_ON_BORDERS_SWAP_MISSET | If this flag is set, enter Alarm state on borders swap misset | |
0x10 - ALARM_FLAGS_STICKING | If this flag is set, only a STOP command can turn all alarms to 0 | |
0x20 - USB_BREAK_RECONNECT | If this flag is set, the USB brake reconnect module will be enabled | |
0x40 - ALARM_WINDING_MISMATCH | If this flag is set, enter Alarm state when windings mismatch | |
0x80 - ALARM_ENGINE_RESPONSE | If this flag is set, enter the Alarm state on response of the engine control action | |
uint8_t | Reserved [7] | Reserved (7 bytes) |
uint16_t | CRC | Checksum |
Description: Read protection settings.
6.2.6.33. Command GSNI¶
Command code (CMD): “gsni” or 0x696E7367.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (28 bytes)
uint32_t | CMD | Command |
uint8_t | SyncInFlags | Input synchronization flags. This is a bit mask for bitwise operations. |
0x1 - SYNCIN_ENABLED | Synchronization in mode is enabled if this flag is set. | |
0x2 - SYNCIN_INVERT | Trigger on falling edge if flag is set, on rising edge otherwise. | |
0x4 - SYNCIN_GOTOPOSITION | The engine is going to the position specified in Position and uPosition if this flag is set. And it is shifting on the Position and uPosition if this flag is unset | |
uint16_t | ClutterTime | Input synchronization pulse dead time (us). |
int32_t | Position | Desired position or shift (full steps) |
int16_t | uPosition | The fractional part of a position or shift in microsteps. It is used with a stepper motor. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
uint32_t | Speed | Target speed (for stepper motor: steps/s, for DC: rpm). Range: 0..100000. |
uint8_t | uSpeed | Target speed in microsteps/s. Microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). Used a stepper motor only. |
uint8_t | Reserved [8] | Reserved (8 bytes) |
uint16_t | CRC | Checksum |
Description: Read input synchronization settings. This function reads the structure with a set of input synchronization settings, modes, periods and flags that specify the behavior of input synchronization. All boards are supplied with the standard set of these settings.
6.2.6.34. Command GSNO¶
Command code (CMD): “gsno” or 0x6F6E7367.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (16 bytes)
uint32_t | CMD | Command |
uint8_t | SyncOutFlags | Output synchronization flags. This is a bit mask for bitwise operations. |
0x1 - SYNCOUT_ENABLED | The synchronization out pin follows the synchronization logic if the flag is set. Otherwise, it is governed by the SYNCOUT_STATE flag. | |
0x2 - SYNCOUT_STATE | When the output state is fixed by the negative SYNCOUT_ENABLED flag, the pin state is in accordance with this flag state. | |
0x4 - SYNCOUT_INVERT | The low level is active if the flag is set. Otherwise, the high level is active. | |
0x8 - SYNCOUT_IN_STEPS | Use motor steps or encoder pulses instead of milliseconds for output pulse generation if the flag is set. | |
0x10 - SYNCOUT_ONSTART | Generate a synchronization pulse when movement starts. | |
0x20 - SYNCOUT_ONSTOP | Generate a synchronization pulse when movement stops. | |
0x40 - SYNCOUT_ONPERIOD | Generate a synchronization pulse every SyncOutPeriod encoder pulses. | |
uint16_t | SyncOutPulseSteps | This value specifies the duration of output pulse. It is measured microseconds when SYNCOUT_IN_STEPS flag is cleared or in encoder pulses or motor steps when SYNCOUT_IN_STEPS is set. |
uint16_t | SyncOutPeriod | This value specifies the number of encoder pulses or steps between two output synchronization pulses when SYNCOUT_ONPERIOD is set. |
uint32_t | Accuracy | This is the neighborhood around the target coordinates, every point in which is treated as the target position. Getting in these points cause the stop impulse. |
uint8_t | uAccuracy | This is the neighborhood around the target coordinates in microsteps (used with a stepper motor only). The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
uint16_t | CRC | Checksum |
Description: Read output synchronization settings. This function reads the structure containing a set of output synchronization settings, modes, periods and flags that specify the behavior of output synchronization. All boards are supplied with the standard set of these settings.
6.2.6.35. Command GSTI¶
Command code (CMD): “gsti” or 0x69747367.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (70 bytes)
uint32_t | CMD | Command |
int8_t | Manufacturer | Manufacturer. Max string length: 16 chars. |
int8_t | PartNumber | Series and PartNumber. Max string length: 24 chars. |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Description: Deprecated. Read stage information from the EEPROM.
6.2.6.36. Command GSTS¶
Command code (CMD): “gsts” or 0x73747367.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (70 bytes)
uint32_t | CMD | Command |
float | LeadScrewPitch | Lead screw pitch (mm). Data type: float. |
int8_t | Units | Units for MaxSpeed and TravelRange fields of the structure (steps, degrees, mm, …). Max string length: 8 chars. |
float | MaxSpeed | Maximum speed (Units/c). Data type: float. |
float | TravelRange | Travel range (Units). Data type: float. |
float | SupplyVoltageMin | Minimum supply voltage (V). Data type: float. |
float | SupplyVoltageMax | Maximum supply voltage (V). Data type: float. |
float | MaxCurrentConsumption | Maximum current consumption (A). Data type: float. |
float | HorizontalLoadCapacity | Horizontal load capacity (kg). Data type: float. |
float | VerticalLoadCapacity | Vertical load capacity (kg). Data type: float. |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Description: Deprecated. Read stage settings from the EEPROM.
6.2.6.37. Command GURT¶
Command code (CMD): “gurt” or 0x74727567.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (16 bytes)
uint32_t | CMD | Command |
uint32_t | Speed | UART baudrate (in bauds) |
uint16_t | UARTSetupFlags | UART setup flags. This is a bit mask for bitwise operations. |
0x3 - UART_PARITY_BITS | Bits of the parity. | |
0x0 - UART_PARITY_BIT_EVEN | Parity bit 1, if even | |
0x1 - UART_PARITY_BIT_ODD | Parity bit 1, if odd | |
0x2 - UART_PARITY_BIT_SPACE | Parity bit always 0 | |
0x3 - UART_PARITY_BIT_MARK | Parity bit always 1 | |
0x4 - UART_PARITY_BIT_USE | None parity | |
0x8 - UART_STOP_BIT | If set - one stop bit, else two stop bit | |
uint8_t | Reserved [4] | Reserved (4 bytes) |
uint16_t | CRC | Checksum |
Description: Read UART settings. This function reads the structure containing UART settings.
6.2.6.38. Command SACC¶
Command code (CMD): “sacc” or 0x63636173.
Request: (114 bytes)
uint32_t | CMD | Command |
int8_t | MagneticBrakeInfo | The manufacturer and the part number of magnetic brake, the maximum string length is 24 characters. |
float | MBRatedVoltage | Rated voltage for controlling the magnetic brake (V). Data type: float. |
float | MBRatedCurrent | Rated current for controlling the magnetic brake (A). Data type: float. |
float | MBTorque | Retention moment (mN m). Data type: float. |
uint32_t | MBSettings | Flags of magnetic brake settings. This is a bit mask for bitwise operations. |
0x1 - MB_AVAILABLE | If the flag is set, the magnetic brake is available | |
0x2 - MB_POWERED_HOLD | If this flag is set, the magnetic brake is on when powered | |
int8_t | TemperatureSensorInfo | The manufacturer and the part number of the temperature sensor, the maximum string length: 24 characters. |
float | TSMin | The minimum measured temperature (degrees Celsius) Data type: float. |
float | TSMax | The maximum measured temperature (degrees Celsius) Data type: float. |
float | TSGrad | The temperature gradient (V/degrees Celsius). Data type: float. |
uint32_t | TSSettings | Flags of temperature sensor settings. This is a bit mask for bitwise operations. |
0x7 - TS_TYPE_BITS | Bits of the temperature sensor type | |
0x0 - TS_TYPE_UNKNOWN | Unknown type of sensor | |
0x1 - TS_TYPE_THERMOCOUPLE | Thermocouple | |
0x2 - TS_TYPE_SEMICONDUCTOR | The semiconductor temperature sensor | |
0x8 - TS_AVAILABLE | If the flag is set, the temperature sensor is available | |
uint32_t | LimitSwitchesSettings | Flags of limit switches settings. This is a bit mask for bitwise operations. |
0x1 - LS_ON_SW1_AVAILABLE | If the flag is set, the limit switch connected to pin SW1 is available | |
0x2 - LS_ON_SW2_AVAILABLE | If the flag is set, the limit switch connected to pin SW2 is available | |
0x4 - LS_SW1_ACTIVE_LOW | If the flag is set, the limit switch connected to pin SW1 is triggered by a low level on the pin | |
0x8 - LS_SW2_ACTIVE_LOW | If the flag is set, the limit switch connected to pin SW2 is triggered by a low level on pin | |
0x10 - LS_SHORTED | If the flag is set, the limit switches are shorted | |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Deprecated. Set additional accessories’ information to the EEPROM. Can be used by the manufacturer only.
6.2.6.39. Command SBRK¶
Command code (CMD): “sbrk” or 0x6B726273.
Request: (25 bytes)
uint32_t | CMD | Command |
uint16_t | t1 | Time in ms between turning on motor power and turning off the brake. |
uint16_t | t2 | Time in ms between the brake turning off and moving readiness. All moving commands will execute after this interval. |
uint16_t | t3 | Time in ms between motor stop and the brake turning on. |
uint16_t | t4 | Time in ms between turning on the brake and turning off motor power. |
uint8_t | BrakeFlags | Flags. This is a bit mask for bitwise operations. |
0x1 - BRAKE_ENABLED | Brake control is enabled if this flag is set. | |
0x2 - BRAKE_ENG_PWROFF | Brake turns the stepper motor power off if this flag is set. | |
uint8_t | Reserved [10] | Reserved (10 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Set brake control settings.
6.2.6.40. Command SCAL¶
Command code (CMD): “scal” or 0x6C616373.
Request: (118 bytes)
uint32_t | CMD | Command |
float | CSS1_A | Scaling factor for the analog measurements of the A winding current. |
float | CSS1_B | Offset for the analog measurements of the A winding current. |
float | CSS2_A | Scaling factor for the analog measurements of the B winding current. |
float | CSS2_B | Offset for the analog measurements of the B winding current. |
float | FullCurrent_A | Scaling factor for the analog measurements of the full current. |
float | FullCurrent_B | Offset for the analog measurements of the full current. |
uint8_t | Reserved [88] | Reserved (88 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Set calibration settings. Manufacturer only. This function sends the structure with calibration settings to the controller’s memory. These settings are used to convert bare ADC values to winding currents in mA and the full current in mA. Parameters are grouped into pairs, XXX_A and XXX_B, representing linear equation coefficients. The first one is the slope, the second one is the constant term. Thus, XXX_Current[mA] = XXX_A[mA/ADC]XXX_ADC_CODE[ADC] + XXX_B[mA].
6.2.6.41. Command SCTL¶
Command code (CMD): “sctl” or 0x6C746373.
Request: (93 bytes)
uint32_t | CMD | Command |
uint32_t | MaxSpeed | Array of speeds (full step) used with the joystick and the button control. Range: 0..100000. |
uint8_t | uMaxSpeed | Array of speeds (in microsteps) used with the joystick and the button control. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
uint16_t | Timeout | Timeout[i] is timeout in ms. After that, max_speed[i+1] is applied. It’s used with the button control only. |
uint16_t | MaxClickTime | Maximum click time (in ms). Until the expiration of this time, the first speed isn’t applied. |
uint16_t | Flags | Control flags. This is a bit mask for bitwise operations. |
0x3 - CONTROL_MODE_BITS | Bits to control the engine by joystick or buttons. | |
0x0 - CONTROL_MODE_OFF | Control is disabled. | |
0x1 - CONTROL_MODE_JOY | Control by joystick. | |
0x2 - CONTROL_MODE_LR | Control by left/right buttons. | |
0x4 - CONTROL_BTN_LEFT_PUSHED_OPEN | Pushed left button corresponds to the open contact if this flag is set. | |
0x8 - CONTROL_BTN_RIGHT_PUSHED_OPEN | Pushed right button corresponds to open contact if this flag is set. | |
int32_t | DeltaPosition | Position Shift (delta) (full step) |
int16_t | uDeltaPosition | Fractional part of the shift in micro steps. It’s used with a stepper motor only. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
uint8_t | Reserved [9] | Reserved (9 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Read motor control settings. In case of CTL_MODE=1, joystick motor control is enabled. In this mode, the joystick is maximally displaced, the engine tends to move at MaxSpeed[i]. i=0 if another value hasn’t been set at the previous usage. To change the speed index ‘i’, use the buttons. In case of CTL_MODE=2, the motor is controlled by the left/right buttons. When you click on the button, the motor starts moving in the appropriate direction at a speed MaxSpeed[0]. After Timeout[i], motor moves at speed MaxSpeed[i+1]. At the transition between MaxSpeed[i] and MaxSpeed[i+1] the motor just accelerates/decelerates as usual.
6.2.6.42. Command SCTP¶
Command code (CMD): “sctp” or 0x70746373.
Request: (18 bytes)
uint32_t | CMD | Command |
uint8_t | CTPMinError | The minimum difference between the SM position in steps and the encoder position that causes the setting of the STATE_CTP_ERROR flag. Measured in steps. |
uint8_t | CTPFlags | This is a bit mask for bitwise operations. |
0x1 - CTP_ENABLED | The position control is enabled if the flag is set. | |
0x2 - CTP_BASE | The position control is based on the revolution sensor if this flag is set; otherwise, it is based on the encoder. | |
0x4 - CTP_ALARM_ON_ERROR | Set ALARM on mismatch if the flag is set. | |
0x8 - REV_SENS_INV | Typically, the sensor is active when it is at 0, and inversion makes active at 1. That is, if you do not invert, it is normal logic - 0 is the activation. | |
0x10 - CTP_ERROR_CORRECTION | Correct errors that appear when slippage occurs if the flag is set. It works only with the encoder. Incompatible with the flag CTP_ALARM_ON_ERROR. | |
uint8_t | Reserved [10] | Reserved (10 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Set control position settings (used with stepper motor only). When controlling the step motor with the encoder (CTP_BASE=0), it is possible to detect the loss of steps. The controller knows the number of steps per revolution (GENG::StepsPerRev) and the encoder resolution (GFBS::IPT). When the control is enabled (CTP_ENABLED is set), the controller stores the current position in the steps of SM and the current position of the encoder. Next, the encoder position is converted into steps at each step, and if the difference between the current position in steps and the encoder position is greater than CTPMinError, the flag STATE_CTP_ERROR is set. Alternatively, the stepper motor may be controlled with the speed sensor (CTP_BASE 1). In this mode, at the active edges of the input clock, the controller stores the current value of steps. Then, at each revolution, the controller checks how many steps have been passed. When the difference is over the CTPMinError, the STATE_CTP_ERROR flag is set.
6.2.6.43. Command SEAS¶
Command code (CMD): “seas” or 0x73616573.
Request: (54 bytes)
uint32_t | CMD | Command |
uint16_t | stepcloseloop_Kw | Mixing ratio of the actual and set speed, range [0, 100], default value 50. |
uint16_t | stepcloseloop_Kp_low | Position feedback in the low-speed zone, range [0, 65535], default value 1000. |
uint16_t | stepcloseloop_Kp_high | Position feedback in the high-speed zone, range [0, 65535], default value 33. |
uint8_t | Reserved [42] | Reserved (42 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Set engine advanced settings.
6.2.6.44. Command SEDS¶
Command code (CMD): “seds” or 0x73646573.
Request: (26 bytes)
uint32_t | CMD | Command |
uint8_t | BorderFlags | Border flags, specify types of borders and motor behavior at borders. This is a bit mask for bitwise operations. |
0x1 - BORDER_IS_ENCODER | Borders are fixed by predetermined encoder values, if set; borders are placed on limit switches, if not set. | |
0x2 - BORDER_STOP_LEFT | The motor should stop on the left border. | |
0x4 - BORDER_STOP_RIGHT | Motor should stop on right border. | |
0x8 - BORDERS_SWAP_MISSET_DETECTION | Motor should stop on both borders. Need to save motor then wrong border settings is set | |
uint8_t | EnderFlags | Flags specify electrical behavior of limit switches like order and pulled positions. This is a bit mask for bitwise operations. |
0x1 - ENDER_SWAP | First limit switch on the right side, if set; otherwise on the left side. | |
0x2 - ENDER_SW1_ACTIVE_LOW | 1 - Limit switch connected to pin SW1 is triggered by a low level on pin. | |
0x4 - ENDER_SW2_ACTIVE_LOW | 1 - Limit switch connected to pin SW2 is triggered by a low level on pin. | |
int32_t | LeftBorder | Left border position, used if BORDER_IS_ENCODER flag is set. |
int16_t | uLeftBorder | Left border position in microsteps (used with stepper motor only). The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
int32_t | RightBorder | Right border position, used if BORDER_IS_ENCODER flag is set. |
int16_t | uRightBorder | Right border position in microsteps. Used with a stepper motor only. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
uint8_t | Reserved [6] | Reserved (6 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Set border and limit switches settings.
6.2.6.45. Command SEIO¶
Command code (CMD): “seio” or 0x6F696573.
Request: (18 bytes)
uint32_t | CMD | Command |
uint8_t | EXTIOSetupFlags | Configuration flags of the external I-O. This is a bit mask for bitwise operations. |
0x1 - EXTIO_SETUP_OUTPUT | EXTIO works as output if the flag is set, works as input otherwise. | |
0x2 - EXTIO_SETUP_INVERT | Interpret EXTIO state inverted if the flag is set. A falling front is treated as an input event and a low logic level as an active state. | |
uint8_t | EXTIOModeFlags | Flags mode settings external I-O. This is a bit mask for bitwise operations. |
0xf - EXTIO_SETUP_MODE_IN_BITS | Bits of the behavior selector when the signal on input goes to the active state. | |
0x0 - EXTIO_SETUP_MODE_IN_NOP | Do nothing. | |
0x1 - EXTIO_SETUP_MODE_IN_STOP | Issue STOP command, ceasing the engine movement. | |
0x2 - EXTIO_SETUP_MODE_IN_PWOF | Issue PWOF command, powering off all engine windings. | |
0x3 - EXTIO_SETUP_MODE_IN_MOVR | Issue MOVR command with last used settings. | |
0x4 - EXTIO_SETUP_MODE_IN_HOME | Issue HOME command. | |
0x5 - EXTIO_SETUP_MODE_IN_ALARM | Set Alarm when the signal goes to the active state. | |
0xf0 - EXTIO_SETUP_MODE_OUT_BITS | Bits of the output behavior selection. | |
0x0 - EXTIO_SETUP_MODE_OUT_OFF | EXTIO pin always set in inactive state. | |
0x10 - EXTIO_SETUP_MODE_OUT_ON | EXTIO pin always set in active state. | |
0x20 - EXTIO_SETUP_MODE_OUT_MOVING | EXTIO pin stays active during moving state. | |
0x30 - EXTIO_SETUP_MODE_OUT_ALARM | EXTIO pin stays active during the alarm state. | |
0x40 - EXTIO_SETUP_MODE_OUT_MOTOR_ON | EXTIO pin stays active when windings are powered. | |
uint8_t | Reserved [10] | Reserved (10 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Set EXTIO settings. This function sends the structure with a set of EXTIO settings to the controller’s memory. By default, input events are signaled through a rising front, and output states are signaled by a high logic state.
6.2.6.46. Command SEMF¶
Command code (CMD): “semf” or 0x666D6573.
Request: (48 bytes)
uint32_t | CMD | Command |
float | L | Motor winding inductance. |
float | R | Motor winding resistance. |
float | Km | Electromechanical ratio of the motor. |
uint8_t | BackEMFFlags | Auto-settings stepper motor flags. This is a bit mask for bitwise operations. |
0x1 - BACK_EMF_INDUCTANCE_AUTO | Flag of auto-detection of inductance of windings of the engine. | |
0x2 - BACK_EMF_RESISTANCE_AUTO | Flag of auto-detection of resistance of windings of the engine. | |
0x4 - BACK_EMF_KM_AUTO | Flag of auto-detection of electromechanical coefficient of the engine. | |
uint8_t | Reserved [29] | Reserved (29 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Set electromechanical coefficients. The settings are different for different stepper motors. Please set new settings when you change the motor.
6.2.6.47. Command SENG¶
Command code (CMD): “seng” or 0x676E6573.
Request: (34 bytes)
uint32_t | CMD | Command |
uint16_t | NomVoltage | Rated voltage in tens of mV. Controller will keep the voltage drop on motor below this value if ENGINE_LIMIT_VOLT flag is set (used with DC only). |
uint16_t | NomCurrent | Rated current (in mA). Controller will keep current consumed by motor below this value if ENGINE_LIMIT_CURR flag is set. Range: 15..8000 |
uint32_t | NomSpeed | Nominal (maximum) speed (in whole steps/s or rpm for DC and stepper motor as a master encoder). Controller will keep motor shaft RPM below this value if ENGINE_LIMIT_RPM flag is set. Range: 1..100000. |
uint8_t | uNomSpeed | The fractional part of a nominal speed in microsteps (is only used with stepper motor). Microstep size and the range of valid values for this field depend on selected step division mode (see MicrostepMode field in engine_settings). |
uint16_t | EngineFlags | Set of flags specify motor shaft movement algorithm and a list of limitations. This is a bit mask for bitwise operations. |
0x1 - ENGINE_REVERSE | Reverse flag. It determines motor shaft rotation direction that corresponds to feedback counts increasing. If not set (default), motor shaft rotation direction under positive voltage corresponds to feedback counts increasing and vice versa. Change it if you see that positive directions on motor and feedback are opposite. | |
0x2 - ENGINE_CURRENT_AS_RMS | Engine current meaning flag. If the flag is unset, then the engine’s current value is interpreted as the maximum amplitude value. If the flag is set, then the engine current value is interpreted as the root-mean-square current value (for stepper) or as the current value calculated from the maximum heat dissipation (BLDC). | |
0x4 - ENGINE_MAX_SPEED | Max speed flag. If it is set, the engine uses the maximum speed achievable with the present engine settings as its nominal speed. | |
0x8 - ENGINE_ANTIPLAY | Play compensation flag. If it is set, the engine makes backlash (play) compensation and reaches the predetermined position accurately at low speed. | |
0x10 - ENGINE_ACCEL_ON | Acceleration enable flag. If it set, motion begins with acceleration and ends with deceleration. | |
0x20 - ENGINE_LIMIT_VOLT | Maximum motor voltage limit enable flag (is only used with DC motor). | |
0x40 - ENGINE_LIMIT_CURR | Maximum motor current limit enable flag (is only used with DC motor). | |
0x80 - ENGINE_LIMIT_RPM | Maximum motor speed limit enable flag. | |
int16_t | Antiplay | Number of pulses or steps for backlash (play) compensation procedure. Used if ENGINE_ANTIPLAY flag is set. |
uint8_t | MicrostepMode | Settings of microstep mode (Used with stepper motor only). the microstep size and the range of valid values for this field depend on the selected step division mode (see MicrostepMode field in engine_settings). This is a bit mask for bitwise operations. |
0x1 - MICROSTEP_MODE_FULL | Full step mode. | |
0x2 - MICROSTEP_MODE_FRAC_2 | 1/2-step mode. | |
0x3 - MICROSTEP_MODE_FRAC_4 | 1/4-step mode. | |
0x4 - MICROSTEP_MODE_FRAC_8 | 1/8-step mode. | |
0x5 - MICROSTEP_MODE_FRAC_16 | 1/16-step mode. | |
0x6 - MICROSTEP_MODE_FRAC_32 | 1/32-step mode. | |
0x7 - MICROSTEP_MODE_FRAC_64 | 1/64-step mode. | |
0x8 - MICROSTEP_MODE_FRAC_128 | 1/128-step mode. | |
0x9 - MICROSTEP_MODE_FRAC_256 | 1/256-step mode. | |
uint16_t | StepsPerRev | Number of full steps per revolution (Used with stepper motor only). Range: 1..65535. |
uint8_t | Reserved [12] | Reserved (12 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Set engine settings. This function sends a structure with a set of engine settings to the controller’s memory. These settings specify the motor shaft movement algorithm, list of limitations and rated characteristics. Use it when you change the motor, encoder, positioner, etc. Please note that wrong engine settings may lead to device malfunction, which can cause irreversible damage to the board.
6.2.6.48. Command SENI¶
Command code (CMD): “seni” or 0x696E6573.
Request: (70 bytes)
uint32_t | CMD | Command |
int8_t | Manufacturer | Manufacturer. Max string length: 16 chars. |
int8_t | PartNumber | Series and PartNumber. Max string length: 24 chars. |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Deprecated. Set encoder information to the EEPROM. Can be used by the manufacturer only.
6.2.6.49. Command SENS¶
Command code (CMD): “sens” or 0x736E6573.
Request: (54 bytes)
uint32_t | CMD | Command |
float | MaxOperatingFrequency | Maximum operation frequency (kHz). Data type: float. |
float | SupplyVoltageMin | Minimum supply voltage (V). Data type: float. |
float | SupplyVoltageMax | Maximum supply voltage (V). Data type: float. |
float | MaxCurrentConsumption | Max current consumption (mA). Data type: float. |
uint32_t | PPR | The number of counts per revolution |
uint32_t | EncoderSettings | Encoder settings flags. This is a bit mask for bitwise operations. |
0x1 - ENCSET_DIFFERENTIAL_OUTPUT | If the flag is set, the encoder has differential output, otherwise single-ended output | |
0x4 - ENCSET_PUSHPULL_OUTPUT | If the flag is set the encoder has push-pull output, otherwise open drain output | |
0x10 - ENCSET_INDEXCHANNEL_PRESENT | If the flag is set, the encoder has an extra indexed channel | |
0x40 - ENCSET_REVOLUTIONSENSOR_PRESENT | If the flag is set, the encoder has the revolution sensor | |
0x100 - ENCSET_REVOLUTIONSENSOR_ACTIVE_HIGH | If the flag is set, the revolution sensor’s active state is high logic state; otherwise, the active state is low logic state | |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Deprecated. Set encoder settings to the EEPROM. Can be used by the manufacturer only.
6.2.6.50. Command SENT¶
Command code (CMD): “sent” or 0x746E6573.
Request: (14 bytes)
uint32_t | CMD | Command |
uint8_t | EngineType | Engine type. This is a bit mask for bitwise operations. |
0x0 - ENGINE_TYPE_NONE | A value that shouldn’t be used. | |
0x1 - ENGINE_TYPE_DC | DC motor. | |
0x2 - ENGINE_TYPE_2DC | 2 DC motors. | |
0x3 - ENGINE_TYPE_STEP | Step motor. | |
0x4 - ENGINE_TYPE_TEST | Duty cycle are fixed. Used only manufacturer. | |
0x5 - ENGINE_TYPE_BRUSHLESS | Brushless motor. | |
uint8_t | DriverType | Driver type. This is a bit mask for bitwise operations. |
0x1 - DRIVER_TYPE_DISCRETE_FET | Driver with discrete FET keys. Default option. | |
0x2 - DRIVER_TYPE_INTEGRATE | Driver with integrated IC. | |
0x3 - DRIVER_TYPE_EXTERNAL | External driver. | |
uint8_t | Reserved [6] | Reserved (6 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Set engine type and driver type.
6.2.6.51. Command SEST¶
Command code (CMD): “sest” or 0x74736573.
Request: (46 bytes)
uint32_t | CMD | Command |
uint16_t | Param1 | |
uint8_t | Reserved [38] | Reserved (38 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Set extended settings. Currently, it is not in use.
6.2.6.52. Command SFBS¶
Command code (CMD): “sfbs” or 0x73626673.
Request: (18 bytes)
uint32_t | CMD | Command |
uint16_t | IPS | The number of encoder counts per shaft revolution. Range: 1..655535. The field is obsolete, it is recommended to write 0 to IPS and use the extended CountsPerTurn field. You may need to update the controller firmware to the latest version. |
uint8_t | FeedbackType | Type of feedback. This is a bit mask for bitwise operations. |
0x1 - FEEDBACK_ENCODER | Feedback by encoder. | |
0x4 - FEEDBACK_EMF | Feedback by EMF. | |
0x5 - FEEDBACK_NONE | Feedback is absent. | |
0x6 - FEEDBACK_ENCODER_MEDIATED | Feedback by encoder mediated by mechanical transmission (for example leadscrew). | |
uint8_t | FeedbackFlags | Flags. This is a bit mask for bitwise operations. |
0x1 - FEEDBACK_ENC_REVERSE | Reverse count of encoder. | |
0xc0 - FEEDBACK_ENC_TYPE_BITS | Bits of the encoder type. | |
0x0 - FEEDBACK_ENC_TYPE_AUTO | Auto detect encoder type. | |
0x40 - FEEDBACK_ENC_TYPE_SINGLE_ENDED | Single-ended encoder. | |
0x80 - FEEDBACK_ENC_TYPE_DIFFERENTIAL | Differential encoder. | |
uint32_t | CountsPerTurn | The number of encoder counts per shaft revolution. Range: 1..4294967295. To use the CountsPerTurn field, write 0 in the IPS field, otherwise the value from the IPS field will be used. |
uint8_t | Reserved [4] | Reserved (4 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Feedback settings.
6.2.6.53. Command SGRI¶
Command code (CMD): “sgri” or 0x69726773.
Request: (70 bytes)
uint32_t | CMD | Command |
int8_t | Manufacturer | Manufacturer. Max string length: 16 chars. |
int8_t | PartNumber | Series and PartNumber. Max string length: 24 chars. |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Deprecated. Set gear information to the EEPROM. Can be used by the manufacturer only.
6.2.6.54. Command SGRS¶
Command code (CMD): “sgrs” or 0x73726773.
Request: (58 bytes)
uint32_t | CMD | Command |
float | ReductionIn | Input reduction coefficient. (Output = (ReductionOut / ReductionIn) Input) Data type: float. |
float | ReductionOut | Output reduction coefficient. (Output = (ReductionOut / ReductionIn) Input) Data type: float. |
float | RatedInputTorque | Maximum continuous torque (N m). Data type: float. |
float | RatedInputSpeed | Maximum speed on the input shaft (rpm). Data type: float. |
float | MaxOutputBacklash | Output backlash of the reduction gear (degree). Data type: float. |
float | InputInertia | Equivalent input gear inertia (g cm2). Data type: float. |
float | Efficiency | Reduction gear efficiency (%). Data type: float. |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Deprecated. Set gear settings to the EEPROM. Can be used by the manufacturer only.
6.2.6.55. Command SHOM¶
Command code (CMD): “shom” or 0x6D6F6873.
Request: (33 bytes)
uint32_t | CMD | Command |
uint32_t | FastHome | Speed used for first motion (full steps). Range: 0..100000. |
uint8_t | uFastHome | Fractional part of the speed for first motion, microsteps. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
uint32_t | SlowHome | Speed used for second motion (full steps). Range: 0..100000. |
uint8_t | uSlowHome | Part of the speed for second motion, microsteps. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
int32_t | HomeDelta | Distance from break point (full steps). |
int16_t | uHomeDelta | Fractional part of the delta distance, microsteps. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
uint16_t | HomeFlags | Set of flags specifies the direction and stopping conditions. This is a bit mask for bitwise operations. |
0x1 - HOME_DIR_FIRST | The flag defines the direction of the 1st motion after execution of the home command. The direction is to the right if the flag is set, and to the left otherwise. | |
0x2 - HOME_DIR_SECOND | The flag defines the direction of the 2nd motion. The direction is to the right if the flag is set, and to the left otherwise. | |
0x4 - HOME_MV_SEC_EN | Use the second phase of calibration to the home position, if set; otherwise the second phase is skipped. | |
0x8 - HOME_HALF_MV | If the flag is set, the stop signals are ignored during the first half-turn of the second movement. | |
0x30 - HOME_STOP_FIRST_BITS | Bits of the first stop selector. | |
0x10 - HOME_STOP_FIRST_REV | First motion stops by revolution sensor. | |
0x20 - HOME_STOP_FIRST_SYN | First motion stops by synchronization input. | |
0x30 - HOME_STOP_FIRST_LIM | First motion stops by limit switch. | |
0xc0 - HOME_STOP_SECOND_BITS | Bits of the second stop selector. | |
0x40 - HOME_STOP_SECOND_REV | Second motion stops by revolution sensor. | |
0x80 - HOME_STOP_SECOND_SYN | Second motion stops by synchronization input. | |
0xc0 - HOME_STOP_SECOND_LIM | Second motion stops by limit switch. | |
0x100 - HOME_USE_FAST | Use the fast algorithm of calibration to the home position, if set; otherwise the traditional algorithm. | |
uint8_t | Reserved [9] | Reserved (9 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Set home settings. This function sends home position structure to the controller’s memory.
6.2.6.56. Command SHSI¶
Command code (CMD): “shsi” or 0x69736873.
Request: (70 bytes)
uint32_t | CMD | Command |
int8_t | Manufacturer | Manufacturer. Max string length: 16 chars. |
int8_t | PartNumber | Series and PartNumber. Max string length: 24 chars. |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Deprecated. Set hall sensor information to the EEPROM. Can be used by the manufacturer only.
6.2.6.57. Command SHSS¶
Command code (CMD): “shss” or 0x73736873.
Request: (50 bytes)
uint32_t | CMD | Command |
float | MaxOperatingFrequency | Maximum operation frequency (kHz). Data type: float. |
float | SupplyVoltageMin | Minimum supply voltage (V). Data type: float. |
float | SupplyVoltageMax | Maximum supply voltage (V). Data type: float. |
float | MaxCurrentConsumption | Maximum current consumption (mA). Data type: float. |
uint32_t | PPR | The number of counts per revolution |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Deprecated. Set hall sensor settings to the EEPROM. Can be used by the manufacturer only.
6.2.6.58. Command SJOY¶
Command code (CMD): “sjoy” or 0x796F6A73.
Request: (22 bytes)
uint32_t | CMD | Command |
uint16_t | JoyLowEnd | Joystick lower end position. Range: 0..10000. |
uint16_t | JoyCenter | Joystick center position. Range: 0..10000. |
uint16_t | JoyHighEnd | Joystick upper end position. Range: 0..10000. |
uint8_t | ExpFactor | Exponential nonlinearity factor. |
uint8_t | DeadZone | Joystick dead zone. |
uint8_t | JoyFlags | Joystick control flags. This is a bit mask for bitwise operations. |
0x1 - JOY_REVERSE | Joystick action is reversed. The joystick deviation to the upper values corresponds to negative speed and vice versa. | |
uint8_t | Reserved [7] | Reserved (7 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Set joystick position. If joystick position falls outside DeadZone limits, a movement begins. The speed is defined by the joystick’s position in the range of the DeadZone limit to the maximum deviation. Joystick positions inside DeadZone limits correspond to zero speed (a soft stop of motion), and positions beyond Low and High limits correspond to MaxSpeed[i] or -MaxSpeed[i] (see command SCTL), where i = 0 by default and can be changed with the left/right buttons (see command SCTL). If the next speed in the list is zero (both integer and microstep parts), the button press is ignored. The first speed in the list shouldn’t be zero. See the Joystick control section on https://doc.xisupport.com for more information.
6.2.6.59. Command SMOV¶
Command code (CMD): “smov” or 0x766F6D73.
Request: (30 bytes)
uint32_t | CMD | Command |
uint32_t | Speed | Target speed (for stepper motor: steps/s, for DC: rpm). Range: 0..100000. |
uint8_t | uSpeed | Target speed in microstep fractions/s. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). Used with a stepper motor only. |
uint16_t | Accel | Motor shaft acceleration, steps/s^2 (stepper motor) or RPM/s (DC). Range: 1..65535. |
uint16_t | Decel | Motor shaft deceleration, steps/s^2 (stepper motor) or RPM/s (DC). Range: 1..65535. |
uint32_t | AntiplaySpeed | Speed in antiplay mode, full steps/s (stepper motor) or RPM (DC). Range: 0..100000. |
uint8_t | uAntiplaySpeed | Speed in antiplay mode, microsteps/s. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). Used with a stepper motor only. |
uint8_t | MoveFlags | Flags that control movement settings. This is a bit mask for bitwise operations. |
0x1 - RPM_DIV_1000 | This flag indicates that the operating speed specified in the command is set in milliRPM. Applicable only for ENCODER feedback mode and only for BLDC motors. | |
uint8_t | Reserved [9] | Reserved (9 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Movement settings set command (speed, acceleration, threshold, etc.).
6.2.6.60. Command SMTI¶
Command code (CMD): “smti” or 0x69746D73.
Request: (70 bytes)
uint32_t | CMD | Command |
int8_t | Manufacturer | Manufacturer. Max string length: 16 chars. |
int8_t | PartNumber | Series and PartNumber. Max string length: 24 chars. |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Deprecated. Set motor information to the EEPROM. Can be used by the manufacturer only.
6.2.6.61. Command SMTS¶
Command code (CMD): “smts” or 0x73746D73.
Request: (112 bytes)
uint32_t | CMD | Command |
uint8_t | MotorType | Motor type. This is a bit mask for bitwise operations. |
0x0 - MOTOR_TYPE_UNKNOWN | Unknown type of engine | |
0x1 - MOTOR_TYPE_STEP | Step engine | |
0x2 - MOTOR_TYPE_DC | DC engine | |
0x3 - MOTOR_TYPE_BLDC | BLDC engine | |
uint8_t | ReservedField | Reserved |
uint16_t | Poles | Number of pole pairs for DC or BLDC motors or number of steps per rotation for stepper motors. |
uint16_t | Phases | Number of phases for BLDC motors. |
float | NominalVoltage | Nominal voltage on winding (B). Data type: float |
float | NominalCurrent | Maximum direct current in winding for DC and BLDC engines, nominal current in windings for stepper motors (A). Data type: float. |
float | NominalSpeed | Not used. Nominal speed (rpm). Used for DC and BLDC engines. Data type: float. |
float | NominalTorque | Nominal torque (mN m). Used for DC and BLDC engines. Data type: float. |
float | NominalPower | Nominal power (W). Used for DC and BLDC engines. Data type: float. |
float | WindingResistance | Resistance of windings for DC engines, of each of two windings for stepper motors, or of each of three windings for BLDC engines (Ohm). Data type: float. |
float | WindingInductance | Inductance of windings for DC engines, inductance of each of two windings for stepper motors, or inductance of each of three windings for BLDC engines (mH). Data type: float. |
float | RotorInertia | Rotor inertia (g cm2). Data type: float. |
float | StallTorque | Torque hold position for a stepper motor or torque at a motionless rotor for other types of engines (mN m). Data type: float. |
float | DetentTorque | Holding torque position with unpowered windings (mN m). Data type: float. |
float | TorqueConstant | Torque constant that determines the proportionality constant between the maximum rotor torque and current flowing in the winding (mN m / A). Used mainly for DC motors. Data type: float. |
float | SpeedConstant | Velocity constant, which determines the value or the amplitude of the induced voltage on the motion of DC or BLDC motors (rpm / V) or stepper motors (steps/s / V). Data type: float. |
float | SpeedTorqueGradient | Speed torque gradient (rpm / mN m). Data type: float. |
float | MechanicalTimeConstant | Mechanical time constant (ms). Data type: float. |
float | MaxSpeed | The maximum speed for stepper motors (steps/s) or DC and BLDC motors (rmp). Data type: float. |
float | MaxCurrent | The maximum current in the winding (A). Data type: float. |
float | MaxCurrentTime | Safe duration of overcurrent in the winding (ms). Data type: float. |
float | NoLoadCurrent | The current consumption in idle mode (A). Used for DC and BLDC motors. Data type: float. |
float | NoLoadSpeed | Idle speed (rpm). Used for DC and BLDC motors. Data type: float. |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Deprecated. Set motor settings to the EEPROM. Can be used by the manufacturer only.
6.2.6.62. Command SNET¶
Command code (CMD): “snet” or 0x74656E73.
Request: (38 bytes)
uint32_t | CMD | Command |
uint8_t | DHCPEnabled | Indicates the method to get the IP-address. It can be either 0 (static) or 1 (DHCP). |
uint8_t | IPv4Address | IP-address of the device in format x.x.x.x. |
uint8_t | SubnetMask | The mask of the subnet in format x.x.x.x. |
uint8_t | DefaultGateway | Default value of the gateway in format x.x.x.x. |
uint8_t | Reserved [19] | Reserved (19 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Set network settings. Manufacturer only. This function sets the desired network settings.
6.2.6.63. Command SNME¶
Command code (CMD): “snme” or 0x656D6E73.
Request: (30 bytes)
uint32_t | CMD | Command |
int8_t | PositionerName | User’s positioner name. It can be set by a user. Max string length: 16 characters. |
uint8_t | Reserved [8] | Reserved (8 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Write the user’s stage name to EEPROM.
6.2.6.64. Command SNMF¶
Command code (CMD): “snmf” or 0x666D6E73.
Request: (30 bytes)
uint32_t | CMD | Command |
int8_t | ControllerName | User controller name. It may be set by the user. Max string length: 16 characters. |
uint8_t | CtrlFlags | Internal controller settings. This is a bit mask for bitwise operations. |
0x1 - EEPROM_PRECEDENCE | If the flag is set, settings from external EEPROM override controller settings. | |
uint8_t | Reserved [7] | Reserved (7 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Write user’s controller name and internal settings to the FRAM.
6.2.6.65. Command SNVM¶
Command code (CMD): “snvm” or 0x6D766E73.
Request: (36 bytes)
uint32_t | CMD | Command |
uint32_t | UserData | User data. It may be set by the user. Each element of the array stores only 32 bits of user data. This is important on systems where an int type contains more than 4 bytes. For example, on all amd64 systems. |
uint8_t | Reserved [2] | Reserved (2 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Write user data into the FRAM.
6.2.6.66. Command SPID¶
Command code (CMD): “spid” or 0x64697073.
Request: (48 bytes)
uint32_t | CMD | Command |
uint16_t | KpU | Proportional gain for voltage PID routine |
uint16_t | KiU | Integral gain for voltage PID routine |
uint16_t | KdU | Differential gain for voltage PID routine |
float | Kpf | Proportional gain for BLDC position PID routine |
float | Kif | Integral gain for BLDC position PID routine |
float | Kdf | Differential gain for BLDC position PID routine |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Set PID settings. This function sends the structure with a set of PID factors to the controller’s memory. These settings specify the behavior of the PID routine for the positioner. These factors are slightly different for different positioners. All boards are supplied with the standard set of PID settings in the controller’s flash memory. Please use it for loading new PID settings when you change positioner. Please note that wrong PID settings lead to device malfunction.
6.2.6.67. Command SPWD¶
Command code (CMD): “spwd” or 0x64777073.
Request: (36 bytes)
uint32_t | CMD | Command |
int8_t | UserPassword | Password for the web-page that the user can change with a USB command or via web-page. |
uint8_t | Reserved [10] | Reserved (10 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Sets the password. Manufacturer only. This function sets the user password for the device’s web-page.
6.2.6.68. Command SPWR¶
Command code (CMD): “spwr” or 0x72777073.
Request: (20 bytes)
uint32_t | CMD | Command |
uint8_t | HoldCurrent | Holding current, as percent of the nominal current. Range: 0..100. |
uint16_t | CurrReductDelay | Time in ms from going to STOP state to the end of current reduction. |
uint16_t | PowerOffDelay | Time in s from going to STOP state to turning power off. |
uint16_t | CurrentSetTime | Time in ms to reach the nominal current. |
uint8_t | PowerFlags | Flags with parameters of power control. This is a bit mask for bitwise operations. |
0x1 - POWER_REDUCT_ENABLED | Current reduction is enabled after CurrReductDelay if this flag is set. | |
0x2 - POWER_OFF_ENABLED | Power off is enabled after PowerOffDelay if this flag is set. | |
0x4 - POWER_SMOOTH_CURRENT | Current ramp-up/down are performed smoothly during current_set_time if this flag is set. | |
uint8_t | Reserved [6] | Reserved (6 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Set settings of step motor power control. Used with a stepper motor only.
6.2.6.69. Command SSEC¶
Command code (CMD): “ssec” or 0x63657373.
Request: (28 bytes)
uint32_t | CMD | Command |
uint16_t | LowUpwrOff | Lower voltage limit to turn off the motor, in tens of mV. |
uint16_t | CriticalIpwr | Maximum motor current which triggers ALARM state, in mA. |
uint16_t | CriticalUpwr | Maximum motor voltage which triggers ALARM state, in tens of mV. |
uint16_t | CriticalT | Maximum temperature, which triggers ALARM state, in tenths of degrees Celsius. |
uint16_t | CriticalIusb | Maximum USB current which triggers ALARM state, in mA. |
uint16_t | CriticalUusb | Maximum USB voltage which triggers ALARM state, in tens of mV. |
uint16_t | MinimumUusb | Minimum USB voltage which triggers ALARM state, in tens of mV. |
uint8_t | Flags | Critical parameter flags. This is a bit mask for bitwise operations. |
0x1 - ALARM_ON_DRIVER_OVERHEATING | If this flag is set, enter the alarm state on the driver overheat signal. | |
0x2 - LOW_UPWR_PROTECTION | If this flag is set, turn off the motor when the voltage is lower than LowUpwrOff. | |
0x4 - H_BRIDGE_ALERT | If this flag is set then turn off the power unit with a signal problem in one of the transistor bridge. | |
0x8 - ALARM_ON_BORDERS_SWAP_MISSET | If this flag is set, enter Alarm state on borders swap misset | |
0x10 - ALARM_FLAGS_STICKING | If this flag is set, only a STOP command can turn all alarms to 0 | |
0x20 - USB_BREAK_RECONNECT | If this flag is set, the USB brake reconnect module will be enabled | |
0x40 - ALARM_WINDING_MISMATCH | If this flag is set, enter Alarm state when windings mismatch | |
0x80 - ALARM_ENGINE_RESPONSE | If this flag is set, enter the Alarm state on response of the engine control action | |
uint8_t | Reserved [7] | Reserved (7 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Set protection settings.
6.2.6.70. Command SSNI¶
Command code (CMD): “ssni” or 0x696E7373.
Request: (28 bytes)
uint32_t | CMD | Command |
uint8_t | SyncInFlags | Input synchronization flags. This is a bit mask for bitwise operations. |
0x1 - SYNCIN_ENABLED | Synchronization in mode is enabled if this flag is set. | |
0x2 - SYNCIN_INVERT | Trigger on falling edge if flag is set, on rising edge otherwise. | |
0x4 - SYNCIN_GOTOPOSITION | The engine is going to the position specified in Position and uPosition if this flag is set. And it is shifting on the Position and uPosition if this flag is unset | |
uint16_t | ClutterTime | Input synchronization pulse dead time (us). |
int32_t | Position | Desired position or shift (full steps) |
int16_t | uPosition | The fractional part of a position or shift in microsteps. It is used with a stepper motor. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
uint32_t | Speed | Target speed (for stepper motor: steps/s, for DC: rpm). Range: 0..100000. |
uint8_t | uSpeed | Target speed in microsteps/s. Microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). Used a stepper motor only. |
uint8_t | Reserved [8] | Reserved (8 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Set input synchronization settings. This function sends the structure with a set of input synchronization settings that specify the behavior of input synchronization to the controller’s memory. All boards are supplied with the standard set of these settings.
6.2.6.71. Command SSNO¶
Command code (CMD): “ssno” or 0x6F6E7373.
Request: (16 bytes)
uint32_t | CMD | Command |
uint8_t | SyncOutFlags | Output synchronization flags. This is a bit mask for bitwise operations. |
0x1 - SYNCOUT_ENABLED | The synchronization out pin follows the synchronization logic if the flag is set. Otherwise, it is governed by the SYNCOUT_STATE flag. | |
0x2 - SYNCOUT_STATE | When the output state is fixed by the negative SYNCOUT_ENABLED flag, the pin state is in accordance with this flag state. | |
0x4 - SYNCOUT_INVERT | The low level is active if the flag is set. Otherwise, the high level is active. | |
0x8 - SYNCOUT_IN_STEPS | Use motor steps or encoder pulses instead of milliseconds for output pulse generation if the flag is set. | |
0x10 - SYNCOUT_ONSTART | Generate a synchronization pulse when movement starts. | |
0x20 - SYNCOUT_ONSTOP | Generate a synchronization pulse when movement stops. | |
0x40 - SYNCOUT_ONPERIOD | Generate a synchronization pulse every SyncOutPeriod encoder pulses. | |
uint16_t | SyncOutPulseSteps | This value specifies the duration of output pulse. It is measured microseconds when SYNCOUT_IN_STEPS flag is cleared or in encoder pulses or motor steps when SYNCOUT_IN_STEPS is set. |
uint16_t | SyncOutPeriod | This value specifies the number of encoder pulses or steps between two output synchronization pulses when SYNCOUT_ONPERIOD is set. |
uint32_t | Accuracy | This is the neighborhood around the target coordinates, every point in which is treated as the target position. Getting in these points cause the stop impulse. |
uint8_t | uAccuracy | This is the neighborhood around the target coordinates in microsteps (used with a stepper motor only). The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Set output synchronization settings. This function sends the structure with a set of output synchronization settings that specify the behavior of output synchronization to the controller’s memory. All boards are supplied with the standard set of these settings.
6.2.6.72. Command SSTI¶
Command code (CMD): “ssti” or 0x69747373.
Request: (70 bytes)
uint32_t | CMD | Command |
int8_t | Manufacturer | Manufacturer. Max string length: 16 chars. |
int8_t | PartNumber | Series and PartNumber. Max string length: 24 chars. |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Deprecated. Set stage information to the EEPROM. Can be used by the manufacturer only.
6.2.6.73. Command SSTS¶
Command code (CMD): “ssts” or 0x73747373.
Request: (70 bytes)
uint32_t | CMD | Command |
float | LeadScrewPitch | Lead screw pitch (mm). Data type: float. |
int8_t | Units | Units for MaxSpeed and TravelRange fields of the structure (steps, degrees, mm, …). Max string length: 8 chars. |
float | MaxSpeed | Maximum speed (Units/c). Data type: float. |
float | TravelRange | Travel range (Units). Data type: float. |
float | SupplyVoltageMin | Minimum supply voltage (V). Data type: float. |
float | SupplyVoltageMax | Maximum supply voltage (V). Data type: float. |
float | MaxCurrentConsumption | Maximum current consumption (A). Data type: float. |
float | HorizontalLoadCapacity | Horizontal load capacity (kg). Data type: float. |
float | VerticalLoadCapacity | Vertical load capacity (kg). Data type: float. |
uint8_t | Reserved [24] | Reserved (24 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Deprecated. Set stage settings to the EEPROM. Can be used by the manufacturer only
6.2.6.74. Command SURT¶
Command code (CMD): “surt” or 0x74727573.
Request: (16 bytes)
uint32_t | CMD | Command |
uint32_t | Speed | UART baudrate (in bauds) |
uint16_t | UARTSetupFlags | UART setup flags. This is a bit mask for bitwise operations. |
0x3 - UART_PARITY_BITS | Bits of the parity. | |
0x0 - UART_PARITY_BIT_EVEN | Parity bit 1, if even | |
0x1 - UART_PARITY_BIT_ODD | Parity bit 1, if odd | |
0x2 - UART_PARITY_BIT_SPACE | Parity bit always 0 | |
0x3 - UART_PARITY_BIT_MARK | Parity bit always 1 | |
0x4 - UART_PARITY_BIT_USE | None parity | |
0x8 - UART_STOP_BIT | If set - one stop bit, else two stop bit | |
uint8_t | Reserved [4] | Reserved (4 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Set UART settings. This function sends the structure with UART settings to the controller’s memory.
6.2.6.75. Command ASIA¶
Command code (CMD): “asia” or 0x61697361.
Request: (22 bytes)
uint32_t | CMD | Command |
int32_t | Position | Desired position or shift (full steps) |
int16_t | uPosition | The fractional part of a position or shift in microsteps. Used with stepper motor only. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
uint32_t | Time | Time period in which you want to achieve the desired position in microseconds. |
uint8_t | Reserved [6] | Reserved (6 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: This command adds one element to the FIFO command buffer. Commands are executed at an input clock pulse. Each synchronization pulse launches the action described in SSNI if the buffer is empty. Otherwise, the pulse launches the first command from the FIFO. In the latter case, the command is erased from the buffer. The number of remaining empty buffer elements can be found in the GETS.
6.2.6.76. Command CLFR¶
Command code (CMD): “clfr” or 0x72666C63.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: The command to clear the controller’s FRAM. The memory is cleared by filling the whole memory bytes with 0x00. After cleaning, the controller restarts. There is no response to this command.
6.2.6.77. Command CONN¶
Command code (CMD): “conn” or 0x6E6E6F63.
Request: (14 bytes)
uint32_t | CMD | Command |
uint8_t | Reserved [8] | Reserved (8 bytes) |
uint16_t | CRC | Checksum |
Answer: (15 bytes)
uint32_t | CMD | Command |
uint8_t | sresult | Command result. |
uint8_t | Reserved [8] | Reserved (8 bytes) |
uint16_t | CRC | Checksum |
Description: Command to open a ISP session (in-system programming) when downloading the firmware. Result = RESULT_OK if the command loader. Result = RESULT_SOFT_ERROR if an error occurred at the time of the command. The Result is not available through the library command_update_firmware(). The function processes it internally.
6.2.6.78. Command DBGR¶
Command code (CMD): “dbgr” or 0x72676264.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (142 bytes)
uint32_t | CMD | Command |
uint8_t | DebugData | Arbitrary debug data. |
uint8_t | Reserved [8] | Reserved (8 bytes) |
uint16_t | CRC | Checksum |
Description: Read data from firmware for debug purpose. Manufacturer only. Its use depends on context, firmware version and previous history.
6.2.6.79. Command DBGW¶
Command code (CMD): “dbgw” or 0x77676264.
Request: (142 bytes)
uint32_t | CMD | Command |
uint8_t | DebugData | Arbitrary debug data. |
uint8_t | Reserved [8] | Reserved (8 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Write data to firmware for debug purpose. Manufacturer only.
6.2.6.80. Command DISC¶
Command code (CMD): “disc” or 0x63736964.
Request: (14 bytes)
uint32_t | CMD | Command |
uint8_t | Reserved [8] | Reserved (8 bytes) |
uint16_t | CRC | Checksum |
Answer: (15 bytes)
uint32_t | CMD | Command |
uint8_t | sresult | Command result. |
uint8_t | Reserved [8] | Reserved (8 bytes) |
uint16_t | CRC | Checksum |
Description: Command to close the ISP session (in-system programming) when loading firmware. Result = RESULT_OK if the command loader. Result = RESULT_HARD_ERROR if a hardware error occurred at the time of the command. Result = RESULT_SOFT_ERROR if a software error occurred at the time of the command. The Result is not available through the library command_update_firmware(). The function processes it internally.
6.2.6.81. Command EERD¶
Command code (CMD): “eerd” or 0x64726565.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Read settings from the stage’s EEPROM to the controller’s RAM. This operation is performed automatically at the connection of the stage with an EEPROM to the controller. Can be used by the manufacturer only.
6.2.6.82. Command EESV¶
Command code (CMD): “eesv” or 0x76736565.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Save settings from the controller’s RAM to the stage’s EEPROM. Can be used by the manufacturer only.
6.2.6.83. Command GBLV¶
Command code (CMD): “gblv” or 0x766C6267.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (10 bytes)
uint32_t | CMD | Command |
uint8_t | Major | Bootloader major version number |
uint8_t | Minor | Bootloader minor version number |
uint16_t | Release | Bootloader release version number |
uint16_t | CRC | Checksum |
Description: Read the controller’s bootloader version.
6.2.6.84. Command GETC¶
Command code (CMD): “getc” or 0x63746567.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (38 bytes)
uint32_t | CMD | Command |
int16_t | WindingVoltageA | In case of a step motor, it contains the voltage across the winding A (in tens of mV); in case of a brushless motor, it contains the voltage on the first coil; in case of a DC motor, it contains the only winding current. |
int16_t | WindingVoltageB | In case of a step motor, it contains the voltage across the winding B (in tens of mV); in case of a brushless motor, it contains the voltage on the second winding; and in case of a DC motor, this field is not used. |
int16_t | WindingVoltageC | In case of a brushless motor, it contains the voltage on the third winding (in tens of mV); in the case of a step motor and a DC motor, the field is not used. |
int16_t | WindingCurrentA | In case of a step motor, it contains the current in the winding A (in mA); in case of a brushless motor, it contains the current in the winding A; and in case of a DC motor, it contains the only winding current. |
int16_t | WindingCurrentB | In case of a step motor, it contains the current in the winding B (in mA); in case of a brushless motor, it contains the current in the winding B; and in case of a DC motor, the field is not used. |
int16_t | WindingCurrentC | In case of a brushless motor, it contains the current in the winding C (in mA); in case of a step motor and a DC motor, the field is not used. |
uint16_t | Pot | Analog input value, dimensionless. Range: 0..10000 |
uint16_t | Joy | The joystick position, dimensionless. Range: 0..10000 |
int16_t | DutyCycle | PWM duty cycle. |
uint8_t | Reserved [14] | Reserved (14 bytes) |
uint16_t | CRC | Checksum |
Description: Return device electrical parameters, useful for charts. A useful function that fills the structure with a snapshot of the controller voltages and currents.
6.2.6.85. Command GETI¶
Command code (CMD): “geti” or 0x69746567.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (36 bytes)
uint32_t | CMD | Command |
int8_t | Manufacturer | Manufacturer |
int8_t | ManufacturerId | Manufacturer id |
int8_t | ProductDescription | Product description |
uint8_t | Major | The major number of the hardware version. |
uint8_t | Minor | The minor number of the hardware version. |
uint16_t | Release | Release version. |
uint8_t | Reserved [12] | Reserved (12 bytes) |
uint16_t | CRC | Checksum |
Description: Return device information. It’s available in the firmware and the bootloader.
6.2.6.86. Command GETM¶
Command code (CMD): “getm” or 0x6D746567.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (216 bytes)
uint32_t | CMD | Command |
int32_t | Speed | Current speed in microsteps per second (whole steps are recalculated considering the current step division mode) or encoder counts per second. |
int32_t | Error | Current error in microsteps per second (whole steps are recalculated considering the current step division mode) or encoder counts per second. |
uint32_t | Length | Length of actual data in buffer. |
uint8_t | Reserved [6] | Reserved (6 bytes) |
uint16_t | CRC | Checksum |
Description: A command to read the data buffer to build a speed graph and a speed error graph. Filling the buffer starts with the command ‘start_measurements’. The buffer holds 25 points; the points are taken with a period of 1 ms. To create a robust system, read data every 20 ms. If the buffer is full, it is recommended to repeat the readings every 5 ms until the buffer again becomes filled with 20 points. To stop measurements just stop reading data. After buffer overflow measurements will stop automatically.
6.2.6.87. Command GETS¶
Command code (CMD): “gets” or 0x73746567.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (54 bytes)
uint32_t | CMD | Command |
uint8_t | MoveSts | Move state. This is a bit mask for bitwise operations. |
0x1 - MOVE_STATE_MOVING | This flag indicates that the controller is trying to move the motor. Don’t use this flag to wait for the completion of the movement command. Use the MVCMD_RUNNING flag from the MvCmdSts field instead. | |
0x2 - MOVE_STATE_TARGET_SPEED | Target speed is reached, if flag set. | |
0x4 - MOVE_STATE_ANTIPLAY | Motor is playing compensation, if flag set. | |
uint8_t | MvCmdSts | Move command state. This is a bit mask for bitwise operations. |
0x3f - MVCMD_NAME_BITS | Move command bit mask. | |
0x0 - MVCMD_UKNWN | Unknown command. | |
0x1 - MVCMD_MOVE | Command move. | |
0x2 - MVCMD_MOVR | Command movr. | |
0x3 - MVCMD_LEFT | Command left. | |
0x4 - MVCMD_RIGHT | Command rigt. | |
0x5 - MVCMD_STOP | Command stop. | |
0x6 - MVCMD_HOME | Command home. | |
0x7 - MVCMD_LOFT | Command loft. | |
0x8 - MVCMD_SSTP | Command soft stop. | |
0x40 - MVCMD_ERROR | Finish state (1 - move command has finished with an error, 0 - move command has finished correctly). This flag makes sense when MVCMD_RUNNING signals movement completion. | |
0x80 - MVCMD_RUNNING | Move command state (0 - move command has finished, 1 - move command is being executed). | |
uint8_t | PWRSts | Power state of the stepper motor (used with stepper motor only). This is a bit mask for bitwise operations. |
0x0 - PWR_STATE_UNKNOWN | Unknown state, should never happen. | |
0x1 - PWR_STATE_OFF | Motor windings are disconnected from the driver. | |
0x3 - PWR_STATE_NORM | Motor windings are powered by nominal current. | |
0x4 - PWR_STATE_REDUCT | Motor windings are powered by reduced current to lower power consumption. | |
0x5 - PWR_STATE_MAX | Motor windings are powered by the maximum current driver can provide at this voltage. | |
uint8_t | EncSts | Encoder state. This is a bit mask for bitwise operations. |
0x0 - ENC_STATE_ABSENT | Encoder is absent. | |
0x1 - ENC_STATE_UNKNOWN | Encoder state is unknown. | |
0x2 - ENC_STATE_MALFUNC | Encoder is connected and malfunctioning. | |
0x3 - ENC_STATE_REVERS | Encoder is connected and operational but counts in other direction. | |
0x4 - ENC_STATE_OK | Encoder is connected and working properly. | |
uint8_t | WindSts | Windings state. This is a bit mask for bitwise operations. |
0x0 - WIND_A_STATE_ABSENT | Winding A is disconnected. | |
0x1 - WIND_A_STATE_UNKNOWN | Winding A state is unknown. | |
0x2 - WIND_A_STATE_MALFUNC | Winding A is short-circuited. | |
0x3 - WIND_A_STATE_OK | Winding A is connected and working properly. | |
0x0 - WIND_B_STATE_ABSENT | Winding B is disconnected. | |
0x10 - WIND_B_STATE_UNKNOWN | Winding B state is unknown. | |
0x20 - WIND_B_STATE_MALFUNC | Winding B is short-circuited. | |
0x30 - WIND_B_STATE_OK | Winding B is connected and working properly. | |
int32_t | CurPosition | Current position. |
int16_t | uCurPosition | Step motor shaft position in microsteps. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). Used with stepper motors only. |
int64_t | EncPosition | Current encoder position. |
int32_t | CurSpeed | Motor shaft speed in steps/s or rpm. |
int16_t | uCurSpeed | Fractional part of motor shaft speed in microsteps. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). Used with stepper motors only. |
int16_t | Ipwr | Engine current, mA. |
int16_t | Upwr | Power supply voltage, tens of mV. |
int16_t | Iusb | USB current, mA. |
int16_t | Uusb | USB voltage, tens of mV. |
int16_t | CurT | Temperature, tenths of degrees Celsius. |
uint32_t | Flags | A set of flags specifies the motor shaft movement algorithm and a list of limitations. This is a bit mask for bitwise operations. |
0x3f - STATE_CONTR | Flags of controller states. | |
0x1 - STATE_ERRC | Command error encountered. The command received is not in the list of controller known commands. The most possible reason is the outdated firmware. | |
0x2 - STATE_ERRD | Data integrity error encountered. The data inside the command and its CRC code do not correspond. Therefore, the data can’t be considered valid. This error may be caused by EMI in the UART/RS232 interface. | |
0x4 - STATE_ERRV | Value error encountered. The values in the command can’t be applied without correction because they fall outside the valid range. Corrected values were used instead of the original ones. | |
0x10 - STATE_EEPROM_CONNECTED | EEPROM with settings is connected. The built-in stage profile is uploaded from the EEPROM memory chip if the EEPROM_PRECEDENCE flag is set, allowing you to connect various stages to the controller with automatic setup. | |
0x20 - STATE_IS_HOMED | Calibration performed. This means that the relative position scale is calibrated against a hardware absolute position sensor, like a limit switch. Drops after loss of calibration, like harsh stops and possibly skipped steps. | |
0x1b3ffc0 - STATE_SECUR | Security flags. | |
0x40 - STATE_ALARM | The controller is in an alarm state, indicating that something dangerous has happened. Most commands are ignored in this state. To reset the flag, a STOP command must be issued. | |
0x80 - STATE_CTP_ERROR | Control position error (is only used with stepper motor). The flag is set when the encoder position and step position are too far apart. | |
0x100 - STATE_POWER_OVERHEAT | Power driver overheat. Motor control is disabled until some cooldown occurs. This should not happen with boxed versions of the controller. This may happen with the bare-board version of the controller with a custom radiator. Redesign your radiator. | |
0x200 - STATE_CONTROLLER_OVERHEAT | Controller overheat. | |
0x400 - STATE_OVERLOAD_POWER_VOLTAGE | Power voltage exceeds safe limit. | |
0x800 - STATE_OVERLOAD_POWER_CURRENT | Power current exceeds safe limit. | |
0x1000 - STATE_OVERLOAD_USB_VOLTAGE | USB voltage exceeds safe limit. | |
0x2000 - STATE_LOW_USB_VOLTAGE | USB voltage is insufficient for normal operation. | |
0x4000 - STATE_OVERLOAD_USB_CURRENT | USB current exceeds safe limit. | |
0x8000 - STATE_BORDERS_SWAP_MISSET | Engine stuck at the wrong edge. | |
0x10000 - STATE_LOW_POWER_VOLTAGE | Power voltage is lower than Low Voltage Protection limit | |
0x20000 - STATE_H_BRIDGE_FAULT | Signal from the driver that fault happened | |
0x100000 - STATE_WINDING_RES_MISMATCH | The difference between winding resistances is too large. This usually happens with a damaged stepper motor with partially short-circuited windings. | |
0x200000 - STATE_ENCODER_FAULT | Signal from the encoder that fault happened | |
0x800000 - STATE_ENGINE_RESPONSE_ERROR | Error response of the engine control action. Motor control algorithm failure means that it can’t make the correct decisions with the feedback data it receives. A single failure may be caused by a mechanical problem. A repeating failure can be caused by incorrect motor settings. | |
0x1000000 - STATE_EXTIO_ALARM | The error is caused by the external EXTIO input signal. | |
uint32_t | GPIOFlags | A set of flags of GPIO states. This is a bit mask for bitwise operations. |
0xffff - STATE_DIG_SIGNAL | Flags of digital signals. | |
0x1 - STATE_RIGHT_EDGE | Engine stuck at the right edge. | |
0x2 - STATE_LEFT_EDGE | Engine stuck at the left edge. | |
0x4 - STATE_BUTTON_RIGHT | Button ‘right’ state (1 if pressed). | |
0x8 - STATE_BUTTON_LEFT | Button ‘left’ state (1 if pressed). | |
0x10 - STATE_GPIO_PINOUT | External GPIO works as out if the flag is set; otherwise, it works as in. | |
0x20 - STATE_GPIO_LEVEL | State of external GPIO pin. | |
0x200 - STATE_BRAKE | State of Brake pin. Flag ‘1’ - if the pin state brake is not powered (brake is clamped), ‘0’ - if the pin state brake is powered (brake is unclamped). | |
0x400 - STATE_REV_SENSOR | State of Revolution sensor pin. | |
0x800 - STATE_SYNC_INPUT | State of Sync input pin. | |
0x1000 - STATE_SYNC_OUTPUT | State of Sync output pin. | |
0x2000 - STATE_ENC_A | State of encoder A pin. | |
0x4000 - STATE_ENC_B | State of encoder B pin. | |
uint8_t | CmdBufFreeSpace | This field is a service field. It shows the number of free synchronization chain buffer cells. |
uint8_t | Reserved [4] | Reserved (4 bytes) |
uint16_t | CRC | Checksum |
Description: Return device state. A useful function that fills the structure with a snapshot of the controller state, including speed, position, and boolean flags.
6.2.6.88. Command GFWV¶
Command code (CMD): “gfwv” or 0x76776667.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (10 bytes)
uint32_t | CMD | Command |
uint8_t | Major | Firmware major version number |
uint8_t | Minor | Firmware minor version number |
uint16_t | Release | Firmware release version number |
uint16_t | CRC | Checksum |
Description: Read the controller’s firmware version.
6.2.6.89. Command GOFW¶
Command code (CMD): “gofw” or 0x77666F67.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (15 bytes)
uint32_t | CMD | Command |
uint8_t | sresult | Result of the command. |
uint8_t | Reserved [8] | Reserved (8 bytes) |
uint16_t | CRC | Checksum |
Description: Command initiates the transfer of control to firmware. This command is also available in the firmware for compatibility. Manufacturer only. Result = RESULT_OK, if the transition from the loader to the firmware is possible. After the response to this command, the transition is executed. Result = RESULT_NO_FIRMWARE if the firmware is not found. Result = RESULT_ALREADY_IN_FIRMWARE if this command is called from the firmware.
6.2.6.90. Command GPOS¶
Command code (CMD): “gpos” or 0x736F7067.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (26 bytes)
uint32_t | CMD | Command |
int32_t | Position | The position of the whole steps in the engine |
int16_t | uPosition | Microstep position is only used with stepper motors. Microstep size and the range of valid values for this field depend on the selected step division mode (see MicrostepMode field in engine_settings). |
int64_t | EncPosition | Encoder position. |
uint8_t | Reserved [6] | Reserved (6 bytes) |
uint16_t | CRC | Checksum |
Description: Reads the value position in steps and microsteps for stepper motor and encoder steps for all engines.
6.2.6.91. Command GSER¶
Command code (CMD): “gser” or 0x72657367.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (10 bytes)
uint32_t | CMD | Command |
uint32_t | SerialNumber | Board serial number. |
uint16_t | CRC | Checksum |
Description: Read device serial number.
6.2.6.92. Command GUID¶
Command code (CMD): “guid” or 0x64697567.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (40 bytes)
uint32_t | CMD | Command |
uint32_t | UniqueID0 | Unique ID 0. |
uint32_t | UniqueID1 | Unique ID 1. |
uint32_t | UniqueID2 | Unique ID 2. |
uint32_t | UniqueID3 | Unique ID 3. |
uint8_t | Reserved [18] | Reserved (18 bytes) |
uint16_t | CRC | Checksum |
Description: This value is unique to each individual device, but is not a random value. Manufacturer only. This unique device identifier can be used to initiate secure boot processes or as a serial number for USB or other end applications.
6.2.6.93. Command HASF¶
Command code (CMD): “hasf” or 0x66736168.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (15 bytes)
uint32_t | CMD | Command |
uint8_t | sresult | Result of command. |
uint8_t | Reserved [8] | Reserved (8 bytes) |
uint16_t | CRC | Checksum |
Description: Check for firmware on device. Manufacturer only. Result = RESULT_NO_FIRMWARE if the firmware is not found. Result = RESULT_HAS_FIRMWARE if the firmware has been found.
6.2.6.94. Command HOME¶
Command code (CMD): “home” or 0x656D6F68.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Moving to home position. Moving algorithm: 1) Moves the motor according to the speed FastHome, uFastHome and flag HOME_DIR_FAST until the limit switch if the HOME_STOP_ENDS flag is set. Or moves the motor until the input synchronization signal occurs if the flag HOME_STOP_SYNC is set. Or moves until the revolution sensor signal occurs if the flag HOME_STOP_REV_SN is set. 2) Then moves according to the speed SlowHome, uSlowHome and flag HOME_DIR_SLOW until the input clock signal occurs if the flag HOME_MV_SEC is set. If the flag HOME_MV_SEC is reset, skip this step. 3) Then shifts the motor according to the speed FastHome, uFastHome and the flag HOME_DIR_SLOW by HomeDelta distance, uHomeDelta. See GHOM/SHOM commands’ description for details on home flags. Moving settings can be set by set_home_settings/set_home_settings_calb.
6.2.6.95. Command IRND¶
Command code (CMD): “irnd” or 0x646E7269.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (24 bytes)
uint32_t | CMD | Command |
uint8_t | key | Random key. |
uint8_t | Reserved [2] | Reserved (2 bytes) |
uint16_t | CRC | Checksum |
Description: Read a random number from the controller. Manufacturer only.
6.2.6.96. Command LEFT¶
Command code (CMD): “left” or 0x7466656C.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Start continuous moving to the left.
6.2.6.97. Command LOFT¶
Command code (CMD): “loft” or 0x74666F6C.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Upon receiving the command ‘loft’, the engine is shifted from the current position to a distance Antiplay defined in engine settings. Then moves to the initial position.
6.2.6.98. Command MOVE¶
Command code (CMD): “move” or 0x65766F6D.
Request: (18 bytes)
uint32_t | CMD | Command |
int32_t | Position | Desired position (full steps or encoder counts). |
int16_t | uPosition | The fractional part of a position in microsteps. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). Used with stepper motor only. |
uint8_t | Reserved [6] | Reserved (6 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Move to position. Upon receiving the command ‘move’ the engine starts to move with pre-set parameters (speed, acceleration, retention), to the point specified by Position and uPosition. uPosition sets the microstep position of a stepper motor. In the case of DC motor, this field is ignored.
6.2.6.99. Command MOVR¶
Command code (CMD): “movr” or 0x72766F6D.
Request: (18 bytes)
uint32_t | CMD | Command |
int32_t | DeltaPosition | Position shift (delta) (full steps or encoder counts) |
int16_t | uDeltaPosition | Fractional part of the shift in microsteps. Used with stepper motor only. The microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
uint8_t | Reserved [6] | Reserved (6 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Shift by a set offset. Upon receiving the command ‘movr’, the engine starts to move with preset parameters (speed, acceleration, hold) left or right (depending on the sign of DeltaPosition). It moves by the number of steps specified in the fields DeltaPosition and uDeltaPosition. uDeltaPosition sets the microstep offset for a stepper motor. In the case of a DC motor, this field is ignored.
6.2.6.100. Command PWOF¶
Command code (CMD): “pwof” or 0x666F7770.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Immediately power off the motor regardless its state. Shouldn’t be used during motion as the motor could be powered on again automatically to continue movement. The command is designed to manually power off the motor. When automatic power off after stop is required, use the power management system.
6.2.6.101. Command RDAN¶
Command code (CMD): “rdan” or 0x6E616472.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (76 bytes)
uint32_t | CMD | Command |
uint16_t | A1Voltage_ADC | ‘Voltage on pin 1 winding A’ raw data from ADC. |
uint16_t | A2Voltage_ADC | ‘Voltage on pin 2 winding A’ raw data from ADC. |
uint16_t | B1Voltage_ADC | ‘Voltage on pin 1 winding B’ raw data from ADC. |
uint16_t | B2Voltage_ADC | ‘Voltage on pin 2 winding B’ raw data from ADC. |
uint16_t | SupVoltage_ADC | ‘Supply voltage of H-bridge’s MOSFETs’ raw data from ADC. |
uint16_t | ACurrent_ADC | ‘Winding A current’ raw data from ADC. |
uint16_t | BCurrent_ADC | ‘Winding B current’ raw data from ADC. |
uint16_t | FullCurrent_ADC | ‘Full current’ raw data from ADC. |
uint16_t | Temp_ADC | Voltage from temperature sensor, raw data from ADC. |
uint16_t | Joy_ADC | Joystick raw data from ADC. |
uint16_t | Pot_ADC | Voltage on analog input, raw data from ADC |
uint16_t | L5_ADC | USB supply voltage after the current sense resistor, raw data from ADC. |
uint16_t | H5_ADC | USB Power supply from ADC |
int16_t | A1Voltage | ‘Voltage on pin 1 winding A’ calibrated data (in tens of mV). |
int16_t | A2Voltage | ‘Voltage on pin 2 winding A’ calibrated data (in tens of mV). |
int16_t | B1Voltage | ‘Voltage on pin 1 winding B’ calibrated data (in tens of mV). |
int16_t | B2Voltage | ‘Voltage on pin 2 winding B’ calibrated data (in tens of mV). |
int16_t | SupVoltage | ‘Supply voltage on the top of H-bridge’s MOSFETs’ calibrated data (in tens of mV). |
int16_t | ACurrent | ‘Winding A current’ calibrated data (in mA). |
int16_t | BCurrent | ‘Winding B current’ calibrated data (in mA). |
int16_t | FullCurrent | ‘Full current’ calibrated data (in mA). |
int16_t | Temp | Temperature, calibrated data (in tenths of degrees Celsius). |
int16_t | Joy | Joystick, calibrated data. Range: 0..10000 |
int16_t | Pot | Analog input, calibrated data. Range: 0..10000 |
int16_t | L5 | USB supply voltage after the current sense resistor (in tens of mV). |
int16_t | H5 | USB power supply (in tens of mV). |
uint16_t | deprecated | |
int32_t | R | Motor winding resistance in mOhms (is only used with stepper motors). |
int32_t | L | Motor winding pseudo inductance in uH (is only used with stepper motors). |
uint8_t | Reserved [8] | Reserved (8 bytes) |
uint16_t | CRC | Checksum |
Description: Read the analog data structure that contains raw analog data from the embedded ADC. This function is used for device testing and deep recalibration by the manufacturer only.
6.2.6.102. Command READ¶
Command code (CMD): “read” or 0x64616572.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Read all settings from the controller’s flash memory to the controller’s RAM, replacing previous data in the RAM.
6.2.6.103. Command RERS¶
Command code (CMD): “rers” or 0x73726572.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Read important settings (calibration coefficients, etc.) from the controller’s flash memory to the controller’s RAM, replacing previous data in the RAM. Manufacturer only.
6.2.6.104. Command REST¶
Command code (CMD): “rest” or 0x74736572.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: The controller reset command. After the reset, the controller goes into bootloader mode. The command is added for compatibility with the loader exchange protocol. There is no response to this command.
6.2.6.105. Command RIGT¶
Command code (CMD): “rigt” or 0x74676972.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Start continuous moving to the right.
6.2.6.106. Command SARS¶
Command code (CMD): “sars” or 0x73726173.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Save important settings (calibration coefficients, etc.) from the controller’s RAM to the controller’s flash memory, replacing previous data in the flash memory. Manufacturer only.
6.2.6.107. Command SAVE¶
Command code (CMD): “save” or 0x65766173.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Save all settings from the controller’s RAM to the controller’s flash memory, replacing previous data in the flash memory.
6.2.6.108. Command SPOS¶
Command code (CMD): “spos” or 0x736F7073.
Request: (26 bytes)
uint32_t | CMD | Command |
int32_t | Position | The position of the whole steps in the engine |
int16_t | uPosition | Microstep position is only used with stepper motors. Microstep size and the range of valid values for this field depend on the selected step division mode (see the MicrostepMode field in engine_settings). |
int64_t | EncPosition | Encoder position. |
uint8_t | PosFlags | Position flags. This is a bit mask for bitwise operations. |
0x1 - SETPOS_IGNORE_POSITION | Will not reload position in steps/microsteps if this flag is set. | |
0x2 - SETPOS_IGNORE_ENCODER | Will not reload encoder state if this flag is set. | |
uint8_t | Reserved [5] | Reserved (5 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Sets position in steps and microsteps for stepper motor. Sets encoder position for all engines.
6.2.6.109. Command SSER¶
Command code (CMD): “sser” or 0x72657373.
Request: (50 bytes)
uint32_t | CMD | Command |
uint32_t | SN | New board serial number. |
uint8_t | Key | Protection key (256 bit). |
uint8_t | Major | The major number of the hardware version. |
uint8_t | Minor | The minor number of the hardware version. |
uint16_t | Release | Number of edits this release of hardware. |
uint8_t | Reserved [4] | Reserved (4 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Write device serial number and hardware version to the controller’s flash memory. Along with the new serial number and hardware version, a ‘Key’ is transmitted. The SN and hardware version are changed and saved when keys match. Can be used by the manufacturer only.
6.2.6.110. Command SSTP¶
Command code (CMD): “sstp” or 0x70747373.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Soft stop the engine. The motor is slowing down with the deceleration specified in move_settings.
6.2.6.111. Command STMS¶
Command code (CMD): “stms” or 0x736D7473.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Start measurements and buffering of speed and the speed error (target speed minus real speed).
6.2.6.112. Command STOP¶
Command code (CMD): “stop” or 0x706F7473.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Immediately stops the engine, moves it to the STOP state, and sets switches to BREAK mode (windings are short-circuited). The holding regime is deactivated for DC motors, keeping current in the windings for stepper motors (to control it, see Power management settings). When this command is called, the ALARM flag is reset.
6.2.6.113. Command UPDF¶
Command code (CMD): “updf” or 0x66647075.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: The command switches the controller to update the firmware state. Manufacturer only. After receiving this command, the firmware board sets a flag (for loader), sends an echo reply, and restarts the controller.
6.2.6.114. Command WDAT¶
Command code (CMD): “wdat” or 0x74616477.
Request: (142 bytes)
uint32_t | CMD | Command |
uint8_t | Data | Encoded firmware. |
uint8_t | Reserved [8] | Reserved (8 bytes) |
uint16_t | CRC | Checksum |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Writes encoded firmware to the controller’s flash memory. The result of each packet write is not available. The overall result is available when the firmware upload is finished.
6.2.6.115. Command WKEY¶
Command code (CMD): “wkey” or 0x79656B77.
Request: (46 bytes)
uint32_t | CMD | Command |
uint8_t | Key | Protection key (256 bit). |
uint8_t | Reserved [8] | Reserved (8 bytes) |
uint16_t | CRC | Checksum |
Answer: (15 bytes)
uint32_t | CMD | Command |
uint8_t | sresult | Command result. |
uint8_t | Reserved [8] | Reserved (8 bytes) |
uint16_t | CRC | Checksum |
Description: Write command key to decrypt the firmware. Result = RESULT_OK, if the command loader. Result = RESULT_HARD_ERROR if there was a mistake at the time of the command. The Result is not available through the library write_key(). The function processes it internally. Can be used by the manufacturer only.
6.2.6.116. Command ZERO¶
Command code (CMD): “zero” or 0x6F72657A.
Request: (4 bytes)
uint32_t | CMD | Command |
Answer: (4 bytes)
uint32_t | CMD | Command |
Description: Sets the current position to 0. Sets the target position of the move command and the movr command to zero for all cases except for movement to the target position. In the latter case, the target position is calculated so that the absolute position of the destination stays the same. For example, if we were at 400 and moved to 500, then the command Zero makes the current position 0 and the position of the destination 100. It does not change the mode of movement. If the motion is carried, it continues, and if the engine is in the ‘hold’, the type of retention remains.
About this document